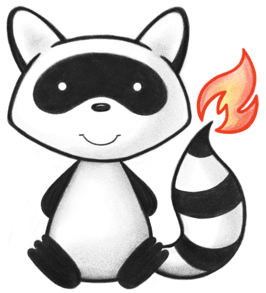
Class FhirRequestBuilder
java.lang.Object
org.hl7.fhir.dstu3.utils.client.network.FhirRequestBuilder
-
Field Summary
Fields -
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionprotected static void
addDefaultHeaders
(okhttp3.Request.Builder request, okhttp3.Headers headers) Adds necessary headers for all REST requests.protected static void
addHeaders
(okhttp3.Request.Builder request, okhttp3.Headers headers) Iterates through the passed inHeaders
and adds them to the providedRequest.Builder
.protected static void
addResourceFormatHeaders
(okhttp3.Request.Builder request, String format) Adds necessary headers for the given resource format provided.protected okhttp3.Request
<T extends Resource>
ResourceRequest<T> execute()
protected static void
formatHeaders
(okhttp3.Request.Builder request, String format, okhttp3.Headers headers) Adds necessary default headers, formatting headers, and any passed inHeaders
to the passed inRequest.Builder
protected okhttp3.OkHttpClient
We only ever want to have one copy of the HttpClient kicking around at any given time.protected static String
getLocationHeader
(okhttp3.Headers headers) Extracts the 'location' header from the passes inHeaders
.protected IParser
Returns the appropriate parser based on the format type passed in.protected static boolean
Returns true if any of theOperationOutcome.OperationOutcomeIssueComponent
within the providedOperationOutcome
have anOperationOutcome.IssueSeverity
ofOperationOutcome.IssueSeverity.ERROR
orOperationOutcome.IssueSeverity.FATAL
protected void
log
(int responseCode, okhttp3.Headers responseHeaders, byte[] responseBody) Logs the givenResponse
, using the currentToolingClientLogger
.protected void
Logs the givenRequest
, using the currentToolingClientLogger
.protected Bundle
unmarshalFeed
(okhttp3.Response response, String format) Unmarshalls Bundle from response stream.protected <T extends Resource>
TunmarshalReference
(okhttp3.Response response, String format) Unmarshalls a resource from the response stream.withHeaders
(okhttp3.Headers headers) withLogger
(org.hl7.fhir.utilities.ToolingClientLogger logger) withMessage
(String message) withResourceFormat
(String resourceFormat) withRetryCount
(int retryCount) withTimeout
(long timeout, TimeUnit unit)
-
Field Details
-
HTTP_PROXY_USER
- See Also:
-
HTTP_PROXY_PASS
- See Also:
-
HEADER_PROXY_AUTH
- See Also:
-
LOCATION_HEADER
- See Also:
-
CONTENT_LOCATION_HEADER
- See Also:
-
DEFAULT_CHARSET
- See Also:
-
-
Constructor Details
-
FhirRequestBuilder
-
-
Method Details
-
formatHeaders
protected static void formatHeaders(okhttp3.Request.Builder request, String format, okhttp3.Headers headers) Adds necessary default headers, formatting headers, and any passed inHeaders
to the passed inRequest.Builder
- Parameters:
request
-Request.Builder
to add headers to.format
- ExpectedResource
format.headers
- Any additionalHeaders
to add to the request.
-
addDefaultHeaders
Adds necessary headers for all REST requests.- User-Agent : hapi-fhir-tooling-client
- Parameters:
request
-Request.Builder
to add default headers to.
-
addResourceFormatHeaders
Adds necessary headers for the given resource format provided.- Parameters:
request
-Request.Builder
to add default headers to.
-
addHeaders
Iterates through the passed inHeaders
and adds them to the providedRequest.Builder
.- Parameters:
request
-Request.Builder
to add headers to.headers
-Headers
to add to request.
-
hasError
Returns true if any of theOperationOutcome.OperationOutcomeIssueComponent
within the providedOperationOutcome
have anOperationOutcome.IssueSeverity
ofOperationOutcome.IssueSeverity.ERROR
orOperationOutcome.IssueSeverity.FATAL
- Parameters:
oo
-OperationOutcome
to evaluate.- Returns:
Boolean.TRUE
if an error exists.
-
getLocationHeader
Extracts the 'location' header from the passes inHeaders
. If no value for 'location' exists, the value for 'content-location' is returned. If neither header exists, we return null.- Parameters:
headers
-Headers
to evaluate- Returns:
String
header value, or null if no location headers are set.
-
getHttpClient
We only ever want to have one copy of the HttpClient kicking around at any given time. If we need to make changes to any configuration, such as proxy settings, timeout, caches, etc, we can do a per-call configuration through theOkHttpClient.newBuilder()
method. That will return a builder that shares the same connection pool, dispatcher, and configuration with the original client. TheOkHttpClient
uses the proxy auth properties set in the current system properties. The reason we don't set the proxy address and authentication explicitly, is due to the fact that this class is often used in conjunction with other http client tools which rely on the system.properties settings to determine proxy settings. It's easier to keep the method consistent across the board. ...for now.- Returns:
OkHttpClient
instance
-
withResourceFormat
-
withHeaders
-
withMessage
-
withRetryCount
-
withLogger
-
withTimeout
-
buildRequest
-
execute
- Throws:
IOException
-
executeAsBatch
- Throws:
IOException
-
unmarshalReference
Unmarshalls a resource from the response stream. -
unmarshalFeed
Unmarshalls Bundle from response stream. -
getParser
Returns the appropriate parser based on the format type passed in. Defaults to XML parser if a blank format is provided...because reasons.Currently supports only "json" and "xml" formats.
- Parameters:
format
- One of "json" or "xml".- Returns:
JsonParser
orXmlParser
-
log
Logs the givenRequest
, using the currentToolingClientLogger
. If the currentlogger
is null, no action is taken.- Parameters:
method
- HTTP request methodurl
- request URLrequestHeaders
-Headers
for requestrequestBody
- Byte array request
-
log
Logs the givenResponse
, using the currentToolingClientLogger
. If the currentlogger
is null, no action is taken.- Parameters:
responseCode
- HTTP response coderesponseHeaders
-Headers
from responseresponseBody
- Byte array response
-