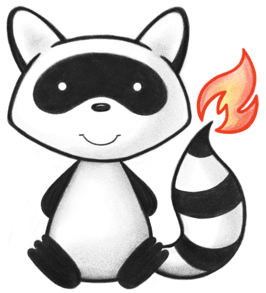
Package org.hl7.fhir.dstu2.model
Class Account
java.lang.Object
org.hl7.fhir.dstu2.model.Base
org.hl7.fhir.dstu2.model.BaseResource
org.hl7.fhir.dstu2.model.Resource
org.hl7.fhir.dstu2.model.DomainResource
org.hl7.fhir.dstu2.model.Account
- All Implemented Interfaces:
ca.uhn.fhir.model.api.IElement
,Serializable
,org.hl7.fhir.instance.model.api.IAnyResource
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseHasExtensions
,org.hl7.fhir.instance.model.api.IBaseHasModifierExtensions
,org.hl7.fhir.instance.model.api.IBaseResource
,org.hl7.fhir.instance.model.api.IDomainResource
A financial tool for tracking value accrued for a particular purpose. In the
healthcare field, used to track charges for a patient, cost centres, etc.
- See Also:
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
static class
-
Field Summary
FieldsModifier and TypeFieldDescriptionprotected Period
Indicates the period of time over which the account is allowed.protected Money
Represents the sum of all credits less all debits associated with the account.protected Period
Identifies the period of time the account applies to; e.g.protected Coding
Identifies the currency to which transactions must be converted when crediting or debiting the account.protected StringType
Provides additional information about what the account tracks and how it is used.protected List
<Identifier> Unique identifier used to reference the account.protected StringType
Name used for the account when displaying it to humans in reports, etc.protected Reference
Indicates the organization, department, etc.protected Organization
The actual object that is the target of the reference (Indicates the organization, department, etc.static final String
static final String
static final String
static final String
static final String
static final String
static final String
static final String
static final String
protected Enumeration
<Account.AccountStatus> Indicates whether the account is presently used/useable or not.protected Reference
Identifies the patient, device, practitioner, location or other object the account is associated with.protected Resource
The actual object that is the target of the reference (Identifies the patient, device, practitioner, location or other object the account is associated with.)protected CodeableConcept
Categorizes the account for reporting and searching purposes.Fields inherited from class org.hl7.fhir.dstu2.model.DomainResource
contained, extension, modifierExtension, text
Fields inherited from class org.hl7.fhir.dstu2.model.Resource
id, implicitRules, language, meta
Fields inherited from interface org.hl7.fhir.instance.model.api.IAnyResource
RES_ID, RES_LAST_UPDATED, RES_PROFILE, RES_SECURITY, RES_TAG, SP_RES_ID, SP_RES_LAST_UPDATED, SP_RES_PROFILE, SP_RES_SECURITY, SP_RES_TAG
Fields inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
INCLUDE_ALL, WILDCARD_ALL_SET
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptioncopy()
boolean
equalsDeep
(Base other) boolean
equalsShallow
(Base other) fhirType()
getName()
getOwner()
getType()
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
hasName()
boolean
boolean
hasOwner()
boolean
boolean
boolean
boolean
hasType()
boolean
isEmpty()
protected void
listChildren
(List<Property> childrenList) setActivePeriod
(Period value) setBalance
(Money value) setCoveragePeriod
(Period value) setCurrency
(Coding value) setDescription
(String value) setDescriptionElement
(StringType value) setNameElement
(StringType value) setOwnerTarget
(Organization value) void
setProperty
(String name, Base value) setStatus
(Account.AccountStatus value) setSubject
(Reference value) setSubjectTarget
(Resource value) setType
(CodeableConcept value) protected Account
Methods inherited from class org.hl7.fhir.dstu2.model.DomainResource
addContained, addExtension, addExtension, addModifierExtension, addModifierExtension, copyValues, getContained, getExtension, getExtensionByUrl, getModifierExtension, getText, hasContained, hasExtension, hasExtension, hasModifierExtension, hasText, setText
Methods inherited from class org.hl7.fhir.dstu2.model.Resource
copyValues, getId, getIdElement, getImplicitRules, getImplicitRulesElement, getLanguage, getLanguageElement, getMeta, hasId, hasIdElement, hasImplicitRules, hasImplicitRulesElement, hasLanguage, hasLanguageElement, hasMeta, setId, setIdElement, setImplicitRules, setImplicitRulesElement, setLanguage, setLanguageElement, setMeta
Methods inherited from class org.hl7.fhir.dstu2.model.BaseResource
getStructureFhirVersionEnum, setId
Methods inherited from class org.hl7.fhir.dstu2.model.Base
castToAddress, castToAnnotation, castToAttachment, castToBase64Binary, castToBoolean, castToCode, castToCodeableConcept, castToCoding, castToContactPoint, castToDate, castToDateTime, castToDecimal, castToDuration, castToElementDefinition, castToExtension, castToHumanName, castToId, castToIdentifier, castToInstant, castToInteger, castToMarkdown, castToMeta, castToMoney, castToNarrative, castToOid, castToPeriod, castToPositiveInt, castToQuantity, castToRange, castToRatio, castToReference, castToResource, castToSampledData, castToSignature, castToSimpleQuantity, castToString, castToTime, castToTiming, castToUnsignedInt, castToUri, children, compareDeep, compareDeep, compareDeep, compareValues, compareValues, equals, getChildByName, getFormatCommentsPost, getFormatCommentsPre, getUserData, getUserInt, getUserString, hasFormatComment, hasType, hasUserData, isMetadataBased, isPrimitive, listChildrenByName, primitiveValue, setUserData, setUserDataINN
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IAnyResource
getId, getIdElement, getLanguageElement, getUserData, setId, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
getFormatCommentsPost, getFormatCommentsPre, hasFormatComment
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
getMeta, getStructureFhirVersionEnum, isDeleted, setId
-
Field Details
-
identifier
Unique identifier used to reference the account. May or may not be intended for human use (e.g. credit card number). -
name
Name used for the account when displaying it to humans in reports, etc. -
type
Categorizes the account for reporting and searching purposes. -
status
Indicates whether the account is presently used/useable or not. -
activePeriod
Indicates the period of time over which the account is allowed. -
currency
Identifies the currency to which transactions must be converted when crediting or debiting the account. -
balance
Represents the sum of all credits less all debits associated with the account. Might be positive, zero or negative. -
coveragePeriod
Identifies the period of time the account applies to; e.g. accounts created per fiscal year, quarter, etc. -
subject
Identifies the patient, device, practitioner, location or other object the account is associated with. -
subjectTarget
The actual object that is the target of the reference (Identifies the patient, device, practitioner, location or other object the account is associated with.) -
owner
Indicates the organization, department, etc. with responsibility for the account. -
ownerTarget
The actual object that is the target of the reference (Indicates the organization, department, etc. with responsibility for the account.) -
description
Provides additional information about what the account tracks and how it is used. -
SP_OWNER
- See Also:
-
SP_IDENTIFIER
- See Also:
-
SP_PERIOD
- See Also:
-
SP_BALANCE
- See Also:
-
SP_SUBJECT
- See Also:
-
SP_PATIENT
- See Also:
-
SP_NAME
- See Also:
-
SP_TYPE
- See Also:
-
SP_STATUS
- See Also:
-
-
Constructor Details
-
Account
public Account()
-
-
Method Details
-
getIdentifier
- Returns:
identifier
(Unique identifier used to reference the account. May or may not be intended for human use (e.g. credit card number).)
-
hasIdentifier
-
addIdentifier
- Returns:
identifier
(Unique identifier used to reference the account. May or may not be intended for human use (e.g. credit card number).)
-
addIdentifier
-
getNameElement
- Returns:
name
(Name used for the account when displaying it to humans in reports, etc.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value
-
hasNameElement
-
hasName
-
setNameElement
- Parameters:
value
-name
(Name used for the account when displaying it to humans in reports, etc.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value
-
getName
- Returns:
- Name used for the account when displaying it to humans in reports, etc.
-
setName
- Parameters:
value
- Name used for the account when displaying it to humans in reports, etc.
-
getType
- Returns:
type
(Categorizes the account for reporting and searching purposes.)
-
hasType
-
setType
- Parameters:
value
-type
(Categorizes the account for reporting and searching purposes.)
-
getStatusElement
- Returns:
status
(Indicates whether the account is presently used/useable or not.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value
-
hasStatusElement
-
hasStatus
-
setStatusElement
- Parameters:
value
-status
(Indicates whether the account is presently used/useable or not.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value
-
getStatus
- Returns:
- Indicates whether the account is presently used/useable or not.
-
setStatus
- Parameters:
value
- Indicates whether the account is presently used/useable or not.
-
getActivePeriod
- Returns:
activePeriod
(Indicates the period of time over which the account is allowed.)
-
hasActivePeriod
-
setActivePeriod
- Parameters:
value
-activePeriod
(Indicates the period of time over which the account is allowed.)
-
getCurrency
- Returns:
currency
(Identifies the currency to which transactions must be converted when crediting or debiting the account.)
-
hasCurrency
-
setCurrency
- Parameters:
value
-currency
(Identifies the currency to which transactions must be converted when crediting or debiting the account.)
-
getBalance
- Returns:
balance
(Represents the sum of all credits less all debits associated with the account. Might be positive, zero or negative.)
-
hasBalance
-
setBalance
- Parameters:
value
-balance
(Represents the sum of all credits less all debits associated with the account. Might be positive, zero or negative.)
-
getCoveragePeriod
- Returns:
coveragePeriod
(Identifies the period of time the account applies to; e.g. accounts created per fiscal year, quarter, etc.)
-
hasCoveragePeriod
-
setCoveragePeriod
- Parameters:
value
-coveragePeriod
(Identifies the period of time the account applies to; e.g. accounts created per fiscal year, quarter, etc.)
-
getSubject
- Returns:
subject
(Identifies the patient, device, practitioner, location or other object the account is associated with.)
-
hasSubject
-
setSubject
- Parameters:
value
-subject
(Identifies the patient, device, practitioner, location or other object the account is associated with.)
-
getSubjectTarget
- Returns:
subject
The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the patient, device, practitioner, location or other object the account is associated with.)
-
setSubjectTarget
- Parameters:
value
-subject
The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the patient, device, practitioner, location or other object the account is associated with.)
-
getOwner
- Returns:
owner
(Indicates the organization, department, etc. with responsibility for the account.)
-
hasOwner
-
setOwner
- Parameters:
value
-owner
(Indicates the organization, department, etc. with responsibility for the account.)
-
getOwnerTarget
- Returns:
owner
The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Indicates the organization, department, etc. with responsibility for the account.)
-
setOwnerTarget
- Parameters:
value
-owner
The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Indicates the organization, department, etc. with responsibility for the account.)
-
getDescriptionElement
- Returns:
description
(Provides additional information about what the account tracks and how it is used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value
-
hasDescriptionElement
-
hasDescription
-
setDescriptionElement
- Parameters:
value
-description
(Provides additional information about what the account tracks and how it is used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value
-
getDescription
- Returns:
- Provides additional information about what the account tracks and how it is used.
-
setDescription
- Parameters:
value
- Provides additional information about what the account tracks and how it is used.
-
listChildren
- Overrides:
listChildren
in classDomainResource
-
setProperty
- Overrides:
setProperty
in classDomainResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
addChild
- Overrides:
addChild
in classDomainResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
fhirType
- Specified by:
fhirType
in interfaceorg.hl7.fhir.instance.model.api.IBase
- Overrides:
fhirType
in classDomainResource
-
copy
- Specified by:
copy
in classDomainResource
-
typedCopy
-
equalsDeep
- Overrides:
equalsDeep
in classDomainResource
-
equalsShallow
- Overrides:
equalsShallow
in classDomainResource
-
isEmpty
- Specified by:
isEmpty
in interfaceorg.hl7.fhir.instance.model.api.IBase
- Overrides:
isEmpty
in classDomainResource
-
getResourceType
- Specified by:
getResourceType
in classResource
-