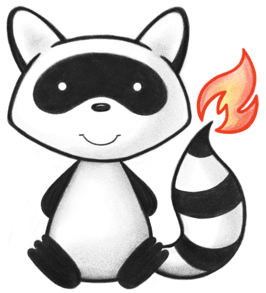
Package org.hl7.fhir.dstu2.model
Class MessageHeader
java.lang.Object
org.hl7.fhir.dstu2.model.Base
org.hl7.fhir.dstu2.model.BaseResource
org.hl7.fhir.dstu2.model.Resource
org.hl7.fhir.dstu2.model.DomainResource
org.hl7.fhir.dstu2.model.MessageHeader
- All Implemented Interfaces:
ca.uhn.fhir.model.api.IElement
,Serializable
,org.hl7.fhir.instance.model.api.IAnyResource
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseHasExtensions
,org.hl7.fhir.instance.model.api.IBaseHasModifierExtensions
,org.hl7.fhir.instance.model.api.IBaseResource
,org.hl7.fhir.instance.model.api.IDomainResource
The header for a message exchange that is either requesting or responding to
an action. The reference(s) that are the subject of the action as well as
other information related to the action are typically transmitted in a bundle
in which the MessageHeader resource instance is the first resource in the
bundle.
- See Also:
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic class
static class
static class
static enum
static class
-
Field Summary
FieldsModifier and TypeFieldDescriptionprotected Reference
The logical author of the message - the person or device that decided the described event should happen.protected Practitioner
The actual object that is the target of the reference (The logical author of the message - the person or device that decided the described event should happen.The actual data of the message - a reference to the root/focus class of the event.The actual objects that are the target of the reference (The actual data of the message - a reference to the root/focus class of the event.)protected List
<MessageHeader.MessageDestinationComponent> The destination application which the message is intended for.protected Reference
The person or device that performed the data entry leading to this message.protected Practitioner
The actual object that is the target of the reference (The person or device that performed the data entry leading to this message.protected Coding
Code that identifies the event this message represents and connects it with its definition.protected CodeableConcept
Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message.protected Reference
Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.protected Resource
The actual object that is the target of the reference (Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.)Information about the message that this message is a response to.protected Reference
The person or organization that accepts overall responsibility for the contents of the message.protected Resource
The actual object that is the target of the reference (The person or organization that accepts overall responsibility for the contents of the message.protected MessageHeader.MessageSourceComponent
The source application from which this message originated.static final String
static final String
static final String
static final String
static final String
static final String
static final String
static final String
static final String
static final String
static final String
static final String
static final String
static final String
protected InstantType
The time that the message was sent.Fields inherited from class org.hl7.fhir.dstu2.model.DomainResource
contained, extension, modifierExtension, text
Fields inherited from class org.hl7.fhir.dstu2.model.Resource
id, implicitRules, language, meta
Fields inherited from interface org.hl7.fhir.instance.model.api.IAnyResource
RES_ID, RES_LAST_UPDATED, RES_PROFILE, RES_SECURITY, RES_TAG, SP_RES_ID, SP_RES_LAST_UPDATED, SP_RES_PROFILE, SP_RES_SECURITY, SP_RES_TAG
Fields inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
INCLUDE_ALL, WILDCARD_ALL_SET
-
Constructor Summary
ConstructorsConstructorDescriptionMessageHeader
(InstantType timestamp, Coding event, MessageHeader.MessageSourceComponent source) -
Method Summary
Modifier and TypeMethodDescriptionaddData()
copy()
boolean
equalsDeep
(Base other) boolean
equalsShallow
(Base other) fhirType()
getData()
getEvent()
boolean
boolean
hasData()
boolean
boolean
boolean
hasEvent()
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
isEmpty()
protected void
listChildren
(List<Property> childrenList) setAuthorTarget
(Practitioner value) setEnterer
(Reference value) setEntererTarget
(Practitioner value) void
setProperty
(String name, Base value) setReason
(CodeableConcept value) setReceiver
(Reference value) setReceiverTarget
(Resource value) setResponsible
(Reference value) setResponsibleTarget
(Resource value) setTimestamp
(Date value) setTimestampElement
(InstantType value) protected MessageHeader
Methods inherited from class org.hl7.fhir.dstu2.model.DomainResource
addContained, addExtension, addExtension, addModifierExtension, addModifierExtension, copyValues, getContained, getExtension, getExtensionByUrl, getModifierExtension, getText, hasContained, hasExtension, hasExtension, hasModifierExtension, hasText, setText
Methods inherited from class org.hl7.fhir.dstu2.model.Resource
copyValues, getId, getIdElement, getImplicitRules, getImplicitRulesElement, getLanguage, getLanguageElement, getMeta, hasId, hasIdElement, hasImplicitRules, hasImplicitRulesElement, hasLanguage, hasLanguageElement, hasMeta, setId, setIdElement, setImplicitRules, setImplicitRulesElement, setLanguage, setLanguageElement, setMeta
Methods inherited from class org.hl7.fhir.dstu2.model.BaseResource
getStructureFhirVersionEnum, setId
Methods inherited from class org.hl7.fhir.dstu2.model.Base
castToAddress, castToAnnotation, castToAttachment, castToBase64Binary, castToBoolean, castToCode, castToCodeableConcept, castToCoding, castToContactPoint, castToDate, castToDateTime, castToDecimal, castToDuration, castToElementDefinition, castToExtension, castToHumanName, castToId, castToIdentifier, castToInstant, castToInteger, castToMarkdown, castToMeta, castToMoney, castToNarrative, castToOid, castToPeriod, castToPositiveInt, castToQuantity, castToRange, castToRatio, castToReference, castToResource, castToSampledData, castToSignature, castToSimpleQuantity, castToString, castToTime, castToTiming, castToUnsignedInt, castToUri, children, compareDeep, compareDeep, compareDeep, compareValues, compareValues, equals, getChildByName, getFormatCommentsPost, getFormatCommentsPre, getUserData, getUserInt, getUserString, hasFormatComment, hasType, hasUserData, isMetadataBased, isPrimitive, listChildrenByName, primitiveValue, setUserData, setUserDataINN
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IAnyResource
getId, getIdElement, getLanguageElement, getUserData, setId, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
getFormatCommentsPost, getFormatCommentsPre, hasFormatComment
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
getMeta, getStructureFhirVersionEnum, isDeleted, setId
-
Field Details
-
timestamp
The time that the message was sent. -
event
Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification have the system value "http://hl7.org/fhir/message-events". -
response
Information about the message that this message is a response to. Only present if this message is a response. -
source
The source application from which this message originated. -
destination
The destination application which the message is intended for. -
enterer
The person or device that performed the data entry leading to this message. Where there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions. -
entererTarget
The actual object that is the target of the reference (The person or device that performed the data entry leading to this message. Where there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions.) -
author
The logical author of the message - the person or device that decided the described event should happen. Where there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions. -
authorTarget
The actual object that is the target of the reference (The logical author of the message - the person or device that decided the described event should happen. Where there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.) -
receiver
Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient. -
receiverTarget
The actual object that is the target of the reference (Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.) -
responsible
The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party. -
responsibleTarget
The actual object that is the target of the reference (The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.) -
reason
Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message. -
data
The actual data of the message - a reference to the root/focus class of the event. -
dataTarget
The actual objects that are the target of the reference (The actual data of the message - a reference to the root/focus class of the event.) -
SP_CODE
- See Also:
-
SP_DATA
- See Also:
-
SP_RECEIVER
- See Also:
-
SP_AUTHOR
- See Also:
-
SP_DESTINATION
- See Also:
-
SP_SOURCE
- See Also:
-
SP_TARGET
- See Also:
-
SP_DESTINATIONURI
- See Also:
-
SP_SOURCEURI
- See Also:
-
SP_RESPONSIBLE
- See Also:
-
SP_RESPONSEID
- See Also:
-
SP_ENTERER
- See Also:
-
SP_EVENT
- See Also:
-
SP_TIMESTAMP
- See Also:
-
-
Constructor Details
-
MessageHeader
public MessageHeader() -
MessageHeader
public MessageHeader(InstantType timestamp, Coding event, MessageHeader.MessageSourceComponent source)
-
-
Method Details
-
getTimestampElement
- Returns:
timestamp
(The time that the message was sent.). This is the underlying object with id, value and extensions. The accessor "getTimestamp" gives direct access to the value
-
hasTimestampElement
-
hasTimestamp
-
setTimestampElement
- Parameters:
value
-timestamp
(The time that the message was sent.). This is the underlying object with id, value and extensions. The accessor "getTimestamp" gives direct access to the value
-
getTimestamp
- Returns:
- The time that the message was sent.
-
setTimestamp
- Parameters:
value
- The time that the message was sent.
-
getEvent
- Returns:
event
(Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification have the system value "http://hl7.org/fhir/message-events".)
-
hasEvent
-
setEvent
- Parameters:
value
-event
(Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification have the system value "http://hl7.org/fhir/message-events".)
-
getResponse
- Returns:
response
(Information about the message that this message is a response to. Only present if this message is a response.)
-
hasResponse
-
setResponse
- Parameters:
value
-response
(Information about the message that this message is a response to. Only present if this message is a response.)
-
getSource
- Returns:
source
(The source application from which this message originated.)
-
hasSource
-
setSource
- Parameters:
value
-source
(The source application from which this message originated.)
-
getDestination
- Returns:
destination
(The destination application which the message is intended for.)
-
hasDestination
-
addDestination
- Returns:
destination
(The destination application which the message is intended for.)
-
addDestination
-
getEnterer
- Returns:
enterer
(The person or device that performed the data entry leading to this message. Where there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions.)
-
hasEnterer
-
setEnterer
- Parameters:
value
-enterer
(The person or device that performed the data entry leading to this message. Where there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions.)
-
getEntererTarget
- Returns:
enterer
The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person or device that performed the data entry leading to this message. Where there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions.)
-
setEntererTarget
- Parameters:
value
-enterer
The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person or device that performed the data entry leading to this message. Where there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions.)
-
getAuthor
- Returns:
author
(The logical author of the message - the person or device that decided the described event should happen. Where there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.)
-
hasAuthor
-
setAuthor
- Parameters:
value
-author
(The logical author of the message - the person or device that decided the described event should happen. Where there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.)
-
getAuthorTarget
- Returns:
author
The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The logical author of the message - the person or device that decided the described event should happen. Where there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.)
-
setAuthorTarget
- Parameters:
value
-author
The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The logical author of the message - the person or device that decided the described event should happen. Where there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.)
-
getReceiver
- Returns:
receiver
(Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.)
-
hasReceiver
-
setReceiver
- Parameters:
value
-receiver
(Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.)
-
getReceiverTarget
- Returns:
receiver
The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.)
-
setReceiverTarget
- Parameters:
value
-receiver
The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.)
-
getResponsible
- Returns:
responsible
(The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.)
-
hasResponsible
-
setResponsible
- Parameters:
value
-responsible
(The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.)
-
getResponsibleTarget
- Returns:
responsible
The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.)
-
setResponsibleTarget
- Parameters:
value
-responsible
The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.)
-
getReason
- Returns:
reason
(Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message.)
-
hasReason
-
setReason
- Parameters:
value
-reason
(Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message.)
-
getData
- Returns:
data
(The actual data of the message - a reference to the root/focus class of the event.)
-
hasData
-
addData
- Returns:
data
(The actual data of the message - a reference to the root/focus class of the event.)
-
addData
-
getDataTarget
- Returns:
data
(The actual objects that are the target of the reference. The reference library doesn't populate this, but you can use this to hold the resources if you resolvethemt. The actual data of the message - a reference to the root/focus class of the event.)
-
listChildren
- Overrides:
listChildren
in classDomainResource
-
setProperty
- Overrides:
setProperty
in classDomainResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
addChild
- Overrides:
addChild
in classDomainResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
fhirType
- Specified by:
fhirType
in interfaceorg.hl7.fhir.instance.model.api.IBase
- Overrides:
fhirType
in classDomainResource
-
copy
- Specified by:
copy
in classDomainResource
-
typedCopy
-
equalsDeep
- Overrides:
equalsDeep
in classDomainResource
-
equalsShallow
- Overrides:
equalsShallow
in classDomainResource
-
isEmpty
- Specified by:
isEmpty
in interfaceorg.hl7.fhir.instance.model.api.IBase
- Overrides:
isEmpty
in classDomainResource
-
getResourceType
- Specified by:
getResourceType
in classResource
-