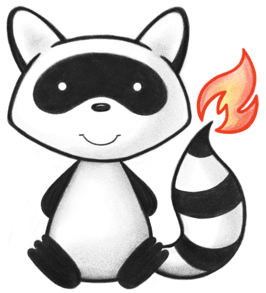
Package org.hl7.fhir.dstu2.model
Class Slot
- All Implemented Interfaces:
ca.uhn.fhir.model.api.IElement
,Serializable
,org.hl7.fhir.instance.model.api.IAnyResource
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseHasExtensions
,org.hl7.fhir.instance.model.api.IBaseHasModifierExtensions
,org.hl7.fhir.instance.model.api.IBaseResource
,org.hl7.fhir.instance.model.api.IDomainResource
A slot of time on a schedule that may be available for booking appointments.
- See Also:
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
static class
-
Field Summary
FieldsModifier and TypeFieldDescriptionprotected StringType
Comments on the slot to describe any extended information.protected InstantType
Date/Time that the slot is to conclude.protected Enumeration
<Slot.SlotStatus> busy | free | busy-unavailable | busy-tentative.protected List
<Identifier> External Ids for this item.protected BooleanType
This slot has already been overbooked, appointments are unlikely to be accepted for this time.protected Reference
The schedule resource that this slot defines an interval of status information.protected Schedule
The actual object that is the target of the reference (The schedule resource that this slot defines an interval of status information.)static final String
static final String
static final String
static final String
static final String
protected InstantType
Date/Time that the slot is to begin.protected CodeableConcept
The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself).Fields inherited from class org.hl7.fhir.dstu2.model.DomainResource
contained, extension, modifierExtension, text
Fields inherited from class org.hl7.fhir.dstu2.model.Resource
id, implicitRules, language, meta
Fields inherited from interface org.hl7.fhir.instance.model.api.IAnyResource
RES_ID, RES_LAST_UPDATED, RES_PROFILE, RES_SECURITY, RES_TAG, SP_RES_ID, SP_RES_LAST_UPDATED, SP_RES_PROFILE, SP_RES_SECURITY, SP_RES_TAG
Fields inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
INCLUDE_ALL, WILDCARD_ALL_SET
-
Constructor Summary
ConstructorsConstructorDescriptionSlot()
Slot
(Reference schedule, Enumeration<Slot.SlotStatus> freeBusyType, InstantType start, InstantType end) -
Method Summary
Modifier and TypeMethodDescriptioncopy()
boolean
equalsDeep
(Base other) boolean
equalsShallow
(Base other) fhirType()
getEnd()
boolean
getStart()
getType()
boolean
boolean
boolean
hasEnd()
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
hasStart()
boolean
boolean
hasType()
boolean
isEmpty()
protected void
listChildren
(List<Property> childrenList) setComment
(String value) setCommentElement
(StringType value) setEndElement
(InstantType value) setFreeBusyType
(Slot.SlotStatus value) setOverbooked
(boolean value) setOverbookedElement
(BooleanType value) void
setProperty
(String name, Base value) setSchedule
(Reference value) setScheduleTarget
(Schedule value) setStartElement
(InstantType value) setType
(CodeableConcept value) protected Slot
Methods inherited from class org.hl7.fhir.dstu2.model.DomainResource
addContained, addExtension, addExtension, addModifierExtension, addModifierExtension, copyValues, getContained, getExtension, getExtensionByUrl, getModifierExtension, getText, hasContained, hasExtension, hasExtension, hasModifierExtension, hasText, setText
Methods inherited from class org.hl7.fhir.dstu2.model.Resource
copyValues, getId, getIdElement, getImplicitRules, getImplicitRulesElement, getLanguage, getLanguageElement, getMeta, hasId, hasIdElement, hasImplicitRules, hasImplicitRulesElement, hasLanguage, hasLanguageElement, hasMeta, setId, setIdElement, setImplicitRules, setImplicitRulesElement, setLanguage, setLanguageElement, setMeta
Methods inherited from class org.hl7.fhir.dstu2.model.BaseResource
getStructureFhirVersionEnum, setId
Methods inherited from class org.hl7.fhir.dstu2.model.Base
castToAddress, castToAnnotation, castToAttachment, castToBase64Binary, castToBoolean, castToCode, castToCodeableConcept, castToCoding, castToContactPoint, castToDate, castToDateTime, castToDecimal, castToDuration, castToElementDefinition, castToExtension, castToHumanName, castToId, castToIdentifier, castToInstant, castToInteger, castToMarkdown, castToMeta, castToMoney, castToNarrative, castToOid, castToPeriod, castToPositiveInt, castToQuantity, castToRange, castToRatio, castToReference, castToResource, castToSampledData, castToSignature, castToSimpleQuantity, castToString, castToTime, castToTiming, castToUnsignedInt, castToUri, children, compareDeep, compareDeep, compareDeep, compareValues, compareValues, equals, getChildByName, getFormatCommentsPost, getFormatCommentsPre, getUserData, getUserInt, getUserString, hasFormatComment, hasType, hasUserData, isMetadataBased, isPrimitive, listChildrenByName, primitiveValue, setUserData, setUserDataINN
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IAnyResource
getId, getIdElement, getLanguageElement, getUserData, setId, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
getFormatCommentsPost, getFormatCommentsPre, hasFormatComment
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
getMeta, getStructureFhirVersionEnum, isDeleted, setId
-
Field Details
-
identifier
External Ids for this item. -
type
The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the availability resource. -
schedule
The schedule resource that this slot defines an interval of status information. -
scheduleTarget
The actual object that is the target of the reference (The schedule resource that this slot defines an interval of status information.) -
freeBusyType
busy | free | busy-unavailable | busy-tentative. -
start
Date/Time that the slot is to begin. -
end
Date/Time that the slot is to conclude. -
overbooked
This slot has already been overbooked, appointments are unlikely to be accepted for this time. -
comment
Comments on the slot to describe any extended information. Such as custom constraints on the slot. -
SP_SCHEDULE
- See Also:
-
SP_IDENTIFIER
- See Also:
-
SP_START
- See Also:
-
SP_SLOTTYPE
- See Also:
-
SP_FBTYPE
- See Also:
-
-
Constructor Details
-
Slot
public Slot() -
Slot
public Slot(Reference schedule, Enumeration<Slot.SlotStatus> freeBusyType, InstantType start, InstantType end)
-
-
Method Details
-
getIdentifier
- Returns:
identifier
(External Ids for this item.)
-
hasIdentifier
-
addIdentifier
- Returns:
identifier
(External Ids for this item.)
-
addIdentifier
-
getType
- Returns:
type
(The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the availability resource.)
-
hasType
-
setType
- Parameters:
value
-type
(The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the availability resource.)
-
getSchedule
- Returns:
schedule
(The schedule resource that this slot defines an interval of status information.)
-
hasSchedule
-
setSchedule
- Parameters:
value
-schedule
(The schedule resource that this slot defines an interval of status information.)
-
getScheduleTarget
- Returns:
schedule
The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The schedule resource that this slot defines an interval of status information.)
-
setScheduleTarget
- Parameters:
value
-schedule
The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The schedule resource that this slot defines an interval of status information.)
-
getFreeBusyTypeElement
- Returns:
freeBusyType
(busy | free | busy-unavailable | busy-tentative.). This is the underlying object with id, value and extensions. The accessor "getFreeBusyType" gives direct access to the value
-
hasFreeBusyTypeElement
-
hasFreeBusyType
-
setFreeBusyTypeElement
- Parameters:
value
-freeBusyType
(busy | free | busy-unavailable | busy-tentative.). This is the underlying object with id, value and extensions. The accessor "getFreeBusyType" gives direct access to the value
-
getFreeBusyType
- Returns:
- busy | free | busy-unavailable | busy-tentative.
-
setFreeBusyType
- Parameters:
value
- busy | free | busy-unavailable | busy-tentative.
-
getStartElement
- Returns:
start
(Date/Time that the slot is to begin.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value
-
hasStartElement
-
hasStart
-
setStartElement
- Parameters:
value
-start
(Date/Time that the slot is to begin.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value
-
getStart
- Returns:
- Date/Time that the slot is to begin.
-
setStart
- Parameters:
value
- Date/Time that the slot is to begin.
-
getEndElement
- Returns:
end
(Date/Time that the slot is to conclude.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value
-
hasEndElement
-
hasEnd
-
setEndElement
- Parameters:
value
-end
(Date/Time that the slot is to conclude.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value
-
getEnd
- Returns:
- Date/Time that the slot is to conclude.
-
setEnd
- Parameters:
value
- Date/Time that the slot is to conclude.
-
getOverbookedElement
- Returns:
overbooked
(This slot has already been overbooked, appointments are unlikely to be accepted for this time.). This is the underlying object with id, value and extensions. The accessor "getOverbooked" gives direct access to the value
-
hasOverbookedElement
-
hasOverbooked
-
setOverbookedElement
- Parameters:
value
-overbooked
(This slot has already been overbooked, appointments are unlikely to be accepted for this time.). This is the underlying object with id, value and extensions. The accessor "getOverbooked" gives direct access to the value
-
getOverbooked
- Returns:
- This slot has already been overbooked, appointments are unlikely to be accepted for this time.
-
setOverbooked
- Parameters:
value
- This slot has already been overbooked, appointments are unlikely to be accepted for this time.
-
getCommentElement
- Returns:
comment
(Comments on the slot to describe any extended information. Such as custom constraints on the slot.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value
-
hasCommentElement
-
hasComment
-
setCommentElement
- Parameters:
value
-comment
(Comments on the slot to describe any extended information. Such as custom constraints on the slot.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value
-
getComment
- Returns:
- Comments on the slot to describe any extended information. Such as custom constraints on the slot.
-
setComment
- Parameters:
value
- Comments on the slot to describe any extended information. Such as custom constraints on the slot.
-
listChildren
- Overrides:
listChildren
in classDomainResource
-
setProperty
- Overrides:
setProperty
in classDomainResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
addChild
- Overrides:
addChild
in classDomainResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
fhirType
- Specified by:
fhirType
in interfaceorg.hl7.fhir.instance.model.api.IBase
- Overrides:
fhirType
in classDomainResource
-
copy
- Specified by:
copy
in classDomainResource
-
typedCopy
-
equalsDeep
- Overrides:
equalsDeep
in classDomainResource
-
equalsShallow
- Overrides:
equalsShallow
in classDomainResource
-
isEmpty
- Specified by:
isEmpty
in interfaceorg.hl7.fhir.instance.model.api.IBase
- Overrides:
isEmpty
in classDomainResource
-
getResourceType
- Specified by:
getResourceType
in classResource
-