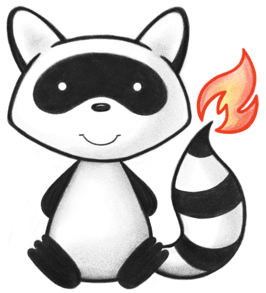
Package org.hl7.fhir.r4.model
Class Address
java.lang.Object
org.hl7.fhir.r4.model.Base
org.hl7.fhir.r4.model.Element
org.hl7.fhir.r4.model.Type
org.hl7.fhir.r4.model.Address
- All Implemented Interfaces:
ca.uhn.fhir.model.api.IElement
,Serializable
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseDatatype
,org.hl7.fhir.instance.model.api.IBaseElement
,org.hl7.fhir.instance.model.api.IBaseHasExtensions
,org.hl7.fhir.instance.model.api.ICompositeType
An address expressed using postal conventions (as opposed to GPS or other
location definition formats). This data type may be used to convey addresses
for use in delivering mail as well as for visiting locations which might not
be valid for mail delivery. There are a variety of postal address formats
defined around the world.
- See Also:
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
static class
static enum
static class
-
Field Summary
FieldsModifier and TypeFieldDescriptionprotected StringType
The name of the city, town, suburb, village or other community or delivery center.protected StringType
Country - a nation as commonly understood or generally accepted.protected StringType
The name of the administrative area (county).protected List
<StringType> This component contains the house number, apartment number, street name, street direction, P.O.protected Period
Time period when address was/is in use.protected StringType
A postal code designating a region defined by the postal service.protected StringType
Sub-unit of a country with limited sovereignty in a federally organized country.protected StringType
Specifies the entire address as it should be displayed e.g.protected Enumeration
<Address.AddressType> Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g.protected Enumeration
<Address.AddressUse> The purpose of this address. -
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptioncopy()
void
copyValues
(Address dst) boolean
equalsDeep
(Base other_) boolean
equalsShallow
(Base other_) fhirType()
getCity()
getLine()
getNamedProperty
(int _hash, String _name, boolean _checkValid) Base[]
getProperty
(int hash, String name, boolean checkValid) getState()
getText()
getType()
String[]
getTypesForProperty
(int hash, String name) getUse()
boolean
hasCity()
boolean
boolean
boolean
boolean
boolean
boolean
hasLine()
boolean
boolean
boolean
boolean
boolean
hasState()
boolean
boolean
hasText()
boolean
boolean
hasType()
boolean
boolean
hasUse()
boolean
boolean
isEmpty()
protected void
listChildren
(List<Property> children) makeProperty
(int hash, String name) void
removeChild
(String name, Base value) setCityElement
(StringType value) setCountry
(String value) setCountryElement
(StringType value) setDistrict
(String value) setDistrictElement
(StringType value) setLine
(List<StringType> theLine) setPostalCode
(String value) setPostalCodeElement
(StringType value) setProperty
(int hash, String name, Base value) setProperty
(String name, Base value) setStateElement
(StringType value) setTextElement
(StringType value) setType
(Address.AddressType value) setUse
(Address.AddressUse value) protected Address
Methods inherited from class org.hl7.fhir.r4.model.Element
addExtension, addExtension, addExtension, copyExtensions, copyNewExtensions, copyValues, getExtension, getExtensionByUrl, getExtensionFirstRep, getExtensionsByUrl, getExtensionsByUrl, getExtensionString, getId, getIdBase, getIdElement, hasExtension, hasExtension, hasExtension, hasExtension, hasId, hasIdElement, isDisallowExtensions, noExtensions, removeExtension, setDisallowExtensions, setExtension, setId, setIdBase, setIdElement
Methods inherited from class org.hl7.fhir.r4.model.Base
castToAddress, castToAnnotation, castToAttachment, castToBase64Binary, castToBoolean, castToCanonical, castToCode, castToCodeableConcept, castToCoding, castToContactDetail, castToContactPoint, castToContributor, castToDataRequirement, castToDate, castToDateTime, castToDecimal, castToDosage, castToDuration, castToElementDefinition, castToExpression, castToExtension, castToHumanName, castToId, castToIdentifier, castToInstant, castToInteger, castToMarkdown, castToMarketingStatus, castToMeta, castToMoney, castToNarrative, castToOid, castToParameterDefinition, castToPeriod, castToPopulation, castToPositiveInt, castToProdCharacteristic, castToProductShelfLife, castToQuantity, castToRange, castToRatio, castToReference, castToRelatedArtifact, castToResource, castToSampledData, castToSignature, castToSimpleQuantity, castToString, castToSubstanceAmount, castToTime, castToTiming, castToTriggerDefinition, castToType, castToUnsignedInt, castToUri, castToUrl, castToUsageContext, castToXhtml, castToXhtmlString, children, clearUserData, compareDeep, compareDeep, compareDeep, compareDeep, compareValues, compareValues, copyValues, dateTimeValue, equals, getChildByName, getChildValueByName, getFormatCommentsPost, getFormatCommentsPre, getNamedProperty, getUserData, getUserInt, getUserString, getXhtml, hasFormatComment, hasPrimitiveValue, hasType, hasUserData, isBooleanPrimitive, isDateTime, isMetadataBased, isPrimitive, isResource, listChildrenByName, listChildrenByName, primitiveValue, setUserData, setUserDataINN
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
getFormatCommentsPost, getFormatCommentsPre, getUserData, hasFormatComment, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseElement
getUserData, setUserData
-
Field Details
-
use
The purpose of this address. -
type
Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both. -
text
Specifies the entire address as it should be displayed e.g. on a postal label. This may be provided instead of or as well as the specific parts. -
line
This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information. -
city
The name of the city, town, suburb, village or other community or delivery center. -
district
The name of the administrative area (county). -
state
Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (e.g. US 2 letter state codes). -
postalCode
A postal code designating a region defined by the postal service. -
country
Country - a nation as commonly understood or generally accepted. -
period
Time period when address was/is in use.
-
-
Constructor Details
-
Address
public Address()Constructor
-
-
Method Details
-
getUseElement
- Returns:
use
(The purpose of this address.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value
-
hasUseElement
-
hasUse
-
setUseElement
- Parameters:
value
-use
(The purpose of this address.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value
-
getUse
- Returns:
- The purpose of this address.
-
setUse
- Parameters:
value
- The purpose of this address.
-
getTypeElement
- Returns:
type
(Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value
-
hasTypeElement
-
hasType
-
setTypeElement
- Parameters:
value
-type
(Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value
-
getType
- Returns:
- Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both.
-
setType
- Parameters:
value
- Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both.
-
getTextElement
- Returns:
text
(Specifies the entire address as it should be displayed e.g. on a postal label. This may be provided instead of or as well as the specific parts.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value
-
hasTextElement
-
hasText
-
setTextElement
- Parameters:
value
-text
(Specifies the entire address as it should be displayed e.g. on a postal label. This may be provided instead of or as well as the specific parts.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value
-
getText
- Returns:
- Specifies the entire address as it should be displayed e.g. on a postal label. This may be provided instead of or as well as the specific parts.
-
setText
- Parameters:
value
- Specifies the entire address as it should be displayed e.g. on a postal label. This may be provided instead of or as well as the specific parts.
-
getLine
- Returns:
line
(This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.)
-
setLine
- Returns:
- Returns a reference to
this
for easy method chaining
-
hasLine
-
addLineElement
- Returns:
line
(This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.)
-
addLine
- Parameters:
value
-line
(This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.)
-
hasLine
- Parameters:
value
-line
(This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.)
-
getCityElement
- Returns:
city
(The name of the city, town, suburb, village or other community or delivery center.). This is the underlying object with id, value and extensions. The accessor "getCity" gives direct access to the value
-
hasCityElement
-
hasCity
-
setCityElement
- Parameters:
value
-city
(The name of the city, town, suburb, village or other community or delivery center.). This is the underlying object with id, value and extensions. The accessor "getCity" gives direct access to the value
-
getCity
- Returns:
- The name of the city, town, suburb, village or other community or delivery center.
-
setCity
- Parameters:
value
- The name of the city, town, suburb, village or other community or delivery center.
-
getDistrictElement
- Returns:
district
(The name of the administrative area (county).). This is the underlying object with id, value and extensions. The accessor "getDistrict" gives direct access to the value
-
hasDistrictElement
-
hasDistrict
-
setDistrictElement
- Parameters:
value
-district
(The name of the administrative area (county).). This is the underlying object with id, value and extensions. The accessor "getDistrict" gives direct access to the value
-
getDistrict
- Returns:
- The name of the administrative area (county).
-
setDistrict
- Parameters:
value
- The name of the administrative area (county).
-
getStateElement
- Returns:
state
(Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (e.g. US 2 letter state codes).). This is the underlying object with id, value and extensions. The accessor "getState" gives direct access to the value
-
hasStateElement
-
hasState
-
setStateElement
- Parameters:
value
-state
(Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (e.g. US 2 letter state codes).). This is the underlying object with id, value and extensions. The accessor "getState" gives direct access to the value
-
getState
- Returns:
- Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (e.g. US 2 letter state codes).
-
setState
- Parameters:
value
- Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (e.g. US 2 letter state codes).
-
getPostalCodeElement
- Returns:
postalCode
(A postal code designating a region defined by the postal service.). This is the underlying object with id, value and extensions. The accessor "getPostalCode" gives direct access to the value
-
hasPostalCodeElement
-
hasPostalCode
-
setPostalCodeElement
- Parameters:
value
-postalCode
(A postal code designating a region defined by the postal service.). This is the underlying object with id, value and extensions. The accessor "getPostalCode" gives direct access to the value
-
getPostalCode
- Returns:
- A postal code designating a region defined by the postal service.
-
setPostalCode
- Parameters:
value
- A postal code designating a region defined by the postal service.
-
getCountryElement
- Returns:
country
(Country - a nation as commonly understood or generally accepted.). This is the underlying object with id, value and extensions. The accessor "getCountry" gives direct access to the value
-
hasCountryElement
-
hasCountry
-
setCountryElement
- Parameters:
value
-country
(Country - a nation as commonly understood or generally accepted.). This is the underlying object with id, value and extensions. The accessor "getCountry" gives direct access to the value
-
getCountry
- Returns:
- Country - a nation as commonly understood or generally accepted.
-
setCountry
- Parameters:
value
- Country - a nation as commonly understood or generally accepted.
-
getPeriod
- Returns:
period
(Time period when address was/is in use.)
-
hasPeriod
-
setPeriod
- Parameters:
value
-period
(Time period when address was/is in use.)
-
listChildren
- Overrides:
listChildren
in classElement
-
getNamedProperty
public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getNamedProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getProperty
public Base[] getProperty(int hash, String name, boolean checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
public Base setProperty(int hash, String name, Base value) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
setProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
- Overrides:
setProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
removeChild
- Overrides:
removeChild
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
makeProperty
- Overrides:
makeProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getTypesForProperty
public String[] getTypesForProperty(int hash, String name) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getTypesForProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
addChild
-
fhirType
-
copy
-
copyValues
-
typedCopy
-
equalsDeep
- Overrides:
equalsDeep
in classElement
-
equalsShallow
- Overrides:
equalsShallow
in classElement
-
isEmpty
-