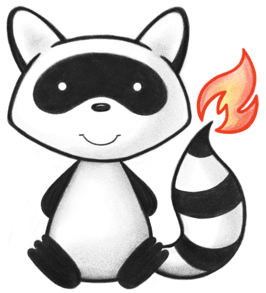
Package org.hl7.fhir.r4.model
Class ParameterDefinition
java.lang.Object
org.hl7.fhir.r4.model.Base
org.hl7.fhir.r4.model.Element
org.hl7.fhir.r4.model.Type
org.hl7.fhir.r4.model.ParameterDefinition
- All Implemented Interfaces:
ca.uhn.fhir.model.api.IElement
,Serializable
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseDatatype
,org.hl7.fhir.instance.model.api.IBaseElement
,org.hl7.fhir.instance.model.api.IBaseHasExtensions
,org.hl7.fhir.instance.model.api.ICompositeType
public class ParameterDefinition
extends Type
implements org.hl7.fhir.instance.model.api.ICompositeType
The parameters to the module. This collection specifies both the input and
output parameters. Input parameters are provided by the caller as part of the
$evaluate operation. Output parameters are included in the GuidanceResponse.
- See Also:
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
static class
-
Field Summary
FieldsModifier and TypeFieldDescriptionprotected StringType
A brief discussion of what the parameter is for and how it is used by the module.protected StringType
The maximum number of times this element is permitted to appear in the request or response.protected IntegerType
The minimum number of times this parameter SHALL appear in the request or response.protected CodeType
The name of the parameter used to allow access to the value of the parameter in evaluation contexts.protected CanonicalType
If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.protected CodeType
The type of the parameter.protected Enumeration
<ParameterDefinition.ParameterUse> Whether the parameter is input or output for the module. -
Constructor Summary
ConstructorsConstructorDescriptionConstructorConstructor -
Method Summary
Modifier and TypeMethodDescriptioncopy()
void
boolean
equalsDeep
(Base other_) boolean
equalsShallow
(Base other_) fhirType()
getMax()
int
getMin()
getName()
getNamedProperty
(int _hash, String _name, boolean _checkValid) Base[]
getProperty
(int hash, String name, boolean checkValid) getType()
String[]
getTypesForProperty
(int hash, String name) getUse()
boolean
boolean
boolean
hasMax()
boolean
boolean
hasMin()
boolean
boolean
hasName()
boolean
boolean
boolean
boolean
hasType()
boolean
boolean
hasUse()
boolean
boolean
isEmpty()
protected void
listChildren
(List<Property> children) makeProperty
(int hash, String name) void
removeChild
(String name, Base value) setDocumentation
(String value) setMaxElement
(StringType value) setMin
(int value) setMinElement
(IntegerType value) setNameElement
(CodeType value) setProfile
(String value) setProfileElement
(CanonicalType value) setProperty
(int hash, String name, Base value) setProperty
(String name, Base value) setTypeElement
(CodeType value) protected ParameterDefinition
Methods inherited from class org.hl7.fhir.r4.model.Element
addExtension, addExtension, addExtension, copyExtensions, copyNewExtensions, copyValues, getExtension, getExtensionByUrl, getExtensionFirstRep, getExtensionsByUrl, getExtensionsByUrl, getExtensionString, getId, getIdBase, getIdElement, hasExtension, hasExtension, hasExtension, hasExtension, hasId, hasIdElement, isDisallowExtensions, noExtensions, removeExtension, setDisallowExtensions, setExtension, setId, setIdBase, setIdElement
Methods inherited from class org.hl7.fhir.r4.model.Base
castToAddress, castToAnnotation, castToAttachment, castToBase64Binary, castToBoolean, castToCanonical, castToCode, castToCodeableConcept, castToCoding, castToContactDetail, castToContactPoint, castToContributor, castToDataRequirement, castToDate, castToDateTime, castToDecimal, castToDosage, castToDuration, castToElementDefinition, castToExpression, castToExtension, castToHumanName, castToId, castToIdentifier, castToInstant, castToInteger, castToMarkdown, castToMarketingStatus, castToMeta, castToMoney, castToNarrative, castToOid, castToParameterDefinition, castToPeriod, castToPopulation, castToPositiveInt, castToProdCharacteristic, castToProductShelfLife, castToQuantity, castToRange, castToRatio, castToReference, castToRelatedArtifact, castToResource, castToSampledData, castToSignature, castToSimpleQuantity, castToString, castToSubstanceAmount, castToTime, castToTiming, castToTriggerDefinition, castToType, castToUnsignedInt, castToUri, castToUrl, castToUsageContext, castToXhtml, castToXhtmlString, children, clearUserData, compareDeep, compareDeep, compareDeep, compareDeep, compareValues, compareValues, copyValues, dateTimeValue, equals, getChildByName, getChildValueByName, getFormatCommentsPost, getFormatCommentsPre, getNamedProperty, getUserData, getUserInt, getUserString, getXhtml, hasFormatComment, hasPrimitiveValue, hasType, hasUserData, isBooleanPrimitive, isDateTime, isMetadataBased, isPrimitive, isResource, listChildrenByName, listChildrenByName, primitiveValue, setUserData, setUserDataINN
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
getFormatCommentsPost, getFormatCommentsPre, getUserData, hasFormatComment, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseElement
getUserData, setUserData
-
Field Details
-
name
The name of the parameter used to allow access to the value of the parameter in evaluation contexts. -
use
Whether the parameter is input or output for the module. -
min
The minimum number of times this parameter SHALL appear in the request or response. -
max
The maximum number of times this element is permitted to appear in the request or response. -
documentation
A brief discussion of what the parameter is for and how it is used by the module. -
type
The type of the parameter. -
profile
If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.
-
-
Constructor Details
-
ParameterDefinition
public ParameterDefinition()Constructor -
ParameterDefinition
Constructor
-
-
Method Details
-
getNameElement
- Returns:
name
(The name of the parameter used to allow access to the value of the parameter in evaluation contexts.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value
-
hasNameElement
-
hasName
-
setNameElement
- Parameters:
value
-name
(The name of the parameter used to allow access to the value of the parameter in evaluation contexts.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value
-
getName
- Returns:
- The name of the parameter used to allow access to the value of the parameter in evaluation contexts.
-
setName
- Parameters:
value
- The name of the parameter used to allow access to the value of the parameter in evaluation contexts.
-
getUseElement
- Returns:
use
(Whether the parameter is input or output for the module.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value
-
hasUseElement
-
hasUse
-
setUseElement
- Parameters:
value
-use
(Whether the parameter is input or output for the module.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value
-
getUse
- Returns:
- Whether the parameter is input or output for the module.
-
setUse
- Parameters:
value
- Whether the parameter is input or output for the module.
-
getMinElement
- Returns:
min
(The minimum number of times this parameter SHALL appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value
-
hasMinElement
-
hasMin
-
setMinElement
- Parameters:
value
-min
(The minimum number of times this parameter SHALL appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value
-
getMin
- Returns:
- The minimum number of times this parameter SHALL appear in the request or response.
-
setMin
- Parameters:
value
- The minimum number of times this parameter SHALL appear in the request or response.
-
getMaxElement
- Returns:
max
(The maximum number of times this element is permitted to appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value
-
hasMaxElement
-
hasMax
-
setMaxElement
- Parameters:
value
-max
(The maximum number of times this element is permitted to appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value
-
getMax
- Returns:
- The maximum number of times this element is permitted to appear in the request or response.
-
setMax
- Parameters:
value
- The maximum number of times this element is permitted to appear in the request or response.
-
getDocumentationElement
- Returns:
documentation
(A brief discussion of what the parameter is for and how it is used by the module.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value
-
hasDocumentationElement
-
hasDocumentation
-
setDocumentationElement
- Parameters:
value
-documentation
(A brief discussion of what the parameter is for and how it is used by the module.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value
-
getDocumentation
- Returns:
- A brief discussion of what the parameter is for and how it is used by the module.
-
setDocumentation
- Parameters:
value
- A brief discussion of what the parameter is for and how it is used by the module.
-
getTypeElement
- Returns:
type
(The type of the parameter.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value
-
hasTypeElement
-
hasType
-
setTypeElement
- Parameters:
value
-type
(The type of the parameter.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value
-
getType
- Returns:
- The type of the parameter.
-
setType
- Parameters:
value
- The type of the parameter.
-
getProfileElement
- Returns:
profile
(If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value
-
hasProfileElement
-
hasProfile
-
setProfileElement
- Parameters:
value
-profile
(If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value
-
getProfile
- Returns:
- If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.
-
setProfile
- Parameters:
value
- If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.
-
listChildren
- Overrides:
listChildren
in classElement
-
getNamedProperty
public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getNamedProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getProperty
public Base[] getProperty(int hash, String name, boolean checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
public Base setProperty(int hash, String name, Base value) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
setProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
- Overrides:
setProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
removeChild
- Overrides:
removeChild
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
makeProperty
- Overrides:
makeProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getTypesForProperty
public String[] getTypesForProperty(int hash, String name) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getTypesForProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
addChild
-
fhirType
-
copy
-
copyValues
-
typedCopy
-
equalsDeep
- Overrides:
equalsDeep
in classElement
-
equalsShallow
- Overrides:
equalsShallow
in classElement
-
isEmpty
-