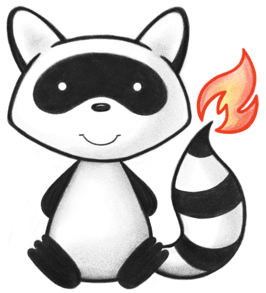
Package org.hl7.fhir.r4.model
Class Quantity
java.lang.Object
org.hl7.fhir.r4.model.Base
org.hl7.fhir.r4.model.Element
org.hl7.fhir.r4.model.Type
org.hl7.fhir.r4.model.Quantity
- All Implemented Interfaces:
ca.uhn.fhir.model.api.IElement
,Serializable
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseDatatype
,org.hl7.fhir.instance.model.api.IBaseElement
,org.hl7.fhir.instance.model.api.IBaseHasExtensions
,org.hl7.fhir.instance.model.api.ICompositeType
,ICoding
- Direct Known Subclasses:
Age
,Count
,Distance
,Duration
,MoneyQuantity
,SimpleQuantity
public class Quantity
extends Type
implements org.hl7.fhir.instance.model.api.ICompositeType, ICoding
A measured amount (or an amount that can potentially be measured). Note that
measured amounts include amounts that are not precisely quantified, including
amounts involving arbitrary units and floating currencies.
- See Also:
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
static class
-
Field Summary
FieldsModifier and TypeFieldDescriptionprotected CodeType
A computer processable form of the unit in some unit representation system.protected Enumeration
<Quantity.QuantityComparator> How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g.protected UriType
The identification of the system that provides the coded form of the unit.protected StringType
A human-readable form of the unit.protected DecimalType
The value of the measured amount. -
Constructor Summary
ConstructorsConstructorDescriptionQuantity()
ConstructorQuantity
(double theValue) Convenience constructorQuantity
(long theValue) Convenience constructorQuantity
(Quantity.QuantityComparator theComparator, double theValue, String theSystem, String theCode, String theUnit) Convenience constructorQuantity
(Quantity.QuantityComparator theComparator, long theValue, String theSystem, String theCode, String theUnit) Convenience constructor -
Method Summary
Modifier and TypeMethodDescriptioncopy()
void
copyValues
(Quantity dst) boolean
equalsDeep
(Base other_) boolean
equalsShallow
(Base other_) fhirType()
static Quantity
getCode()
getNamedProperty
(int _hash, String _name, boolean _checkValid) Base[]
getProperty
(int hash, String name, boolean checkValid) String[]
getTypesForProperty
(int hash, String name) getUnit()
getValue()
boolean
hasCode()
boolean
boolean
boolean
boolean
boolean
boolean
boolean
hasUnit()
boolean
boolean
hasValue()
boolean
boolean
boolean
isEmpty()
protected void
listChildren
(List<Property> children) makeProperty
(int hash, String name) void
removeChild
(String name, Base value) setCodeElement
(CodeType value) setProperty
(int hash, String name, Base value) setProperty
(String name, Base value) setSystemElement
(UriType value) setUnitElement
(StringType value) setValue
(double value) setValue
(long value) setValue
(BigDecimal value) setValueElement
(DecimalType value) boolean
boolean
protected Quantity
Methods inherited from class org.hl7.fhir.r4.model.Element
addExtension, addExtension, addExtension, copyExtensions, copyNewExtensions, copyValues, getExtension, getExtensionByUrl, getExtensionFirstRep, getExtensionsByUrl, getExtensionsByUrl, getExtensionString, getId, getIdBase, getIdElement, hasExtension, hasExtension, hasExtension, hasExtension, hasId, hasIdElement, isDisallowExtensions, noExtensions, removeExtension, setDisallowExtensions, setExtension, setId, setIdBase, setIdElement
Methods inherited from class org.hl7.fhir.r4.model.Base
castToAddress, castToAnnotation, castToAttachment, castToBase64Binary, castToBoolean, castToCanonical, castToCode, castToCodeableConcept, castToCoding, castToContactDetail, castToContactPoint, castToContributor, castToDataRequirement, castToDate, castToDateTime, castToDecimal, castToDosage, castToDuration, castToElementDefinition, castToExpression, castToExtension, castToHumanName, castToId, castToIdentifier, castToInstant, castToInteger, castToMarkdown, castToMarketingStatus, castToMeta, castToMoney, castToNarrative, castToOid, castToParameterDefinition, castToPeriod, castToPopulation, castToPositiveInt, castToProdCharacteristic, castToProductShelfLife, castToQuantity, castToRange, castToRatio, castToReference, castToRelatedArtifact, castToResource, castToSampledData, castToSignature, castToSimpleQuantity, castToString, castToSubstanceAmount, castToTime, castToTiming, castToTriggerDefinition, castToType, castToUnsignedInt, castToUri, castToUrl, castToUsageContext, castToXhtml, castToXhtmlString, children, clearUserData, compareDeep, compareDeep, compareDeep, compareDeep, compareValues, compareValues, copyValues, dateTimeValue, equals, getChildByName, getFormatCommentsPost, getFormatCommentsPre, getNamedProperty, getUserData, getUserInt, getUserString, getXhtml, hasFormatComment, hasPrimitiveValue, hasType, hasUserData, isBooleanPrimitive, isDateTime, isMetadataBased, isPrimitive, isResource, listChildrenByName, listChildrenByName, primitiveValue, setUserData, setUserDataINN
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
getFormatCommentsPost, getFormatCommentsPre, getUserData, hasFormatComment, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseElement
getUserData, setUserData
-
Field Details
-
value
The value of the measured amount. The value includes an implicit precision in the presentation of the value. -
comparator
How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is "invalid input: '<'" , then the real value is invalid input: '<' stated value. -
unit
A human-readable form of the unit. -
system
The identification of the system that provides the coded form of the unit. -
code
A computer processable form of the unit in some unit representation system.
-
-
Constructor Details
-
Quantity
public Quantity()Constructor -
Quantity
Convenience constructor- Parameters:
theValue
- Thevalue
-
Quantity
Convenience constructor- Parameters:
theValue
- Thevalue
-
Quantity
public Quantity(Quantity.QuantityComparator theComparator, double theValue, String theSystem, String theCode, String theUnit) Convenience constructor- Parameters:
theComparator
- Thecomparator
theValue
- Thevalue
theSystem
- ThesetSystem(String)
(the code system for the units}theCode
- ThesetCode(String)
(the code for the units}theUnit
- ThesetUnit(String)
(the human readable display name for the units}
-
Quantity
public Quantity(Quantity.QuantityComparator theComparator, long theValue, String theSystem, String theCode, String theUnit) Convenience constructor- Parameters:
theComparator
- Thecomparator
theValue
- Thevalue
theSystem
- ThesetSystem(String)
(the code system for the units}theCode
- ThesetCode(String)
(the code for the units}theUnit
- ThesetUnit(String)
(the human readable display name for the units}
-
-
Method Details
-
getValueElement
- Returns:
value
(The value of the measured amount. The value includes an implicit precision in the presentation of the value.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value
-
hasValueElement
-
hasValue
-
setValueElement
- Parameters:
value
-value
(The value of the measured amount. The value includes an implicit precision in the presentation of the value.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value
-
getValue
- Returns:
- The value of the measured amount. The value includes an implicit precision in the presentation of the value.
-
setValue
- Parameters:
value
- The value of the measured amount. The value includes an implicit precision in the presentation of the value.
-
setValue
- Parameters:
value
- The value of the measured amount. The value includes an implicit precision in the presentation of the value.
-
setValue
- Parameters:
value
- The value of the measured amount. The value includes an implicit precision in the presentation of the value.
-
getComparatorElement
- Returns:
comparator
(How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is "invalid input: '<'" , then the real value is invalid input: '<' stated value.). This is the underlying object with id, value and extensions. The accessor "getComparator" gives direct access to the value
-
hasComparatorElement
-
hasComparator
-
setComparatorElement
- Parameters:
value
-comparator
(How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is "invalid input: '<'" , then the real value is invalid input: '<' stated value.). This is the underlying object with id, value and extensions. The accessor "getComparator" gives direct access to the value
-
getComparator
- Returns:
- How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is "invalid input: '<'" , then the real value is invalid input: '<' stated value.
-
setComparator
- Parameters:
value
- How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is "invalid input: '<'" , then the real value is invalid input: '<' stated value.
-
getUnitElement
- Returns:
unit
(A human-readable form of the unit.). This is the underlying object with id, value and extensions. The accessor "getUnit" gives direct access to the value
-
hasUnitElement
-
hasUnit
-
setUnitElement
- Parameters:
value
-unit
(A human-readable form of the unit.). This is the underlying object with id, value and extensions. The accessor "getUnit" gives direct access to the value
-
getUnit
- Returns:
- A human-readable form of the unit.
-
setUnit
- Parameters:
value
- A human-readable form of the unit.
-
getSystemElement
- Returns:
system
(The identification of the system that provides the coded form of the unit.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value
-
hasSystemElement
-
hasSystem
-
setSystemElement
- Parameters:
value
-system
(The identification of the system that provides the coded form of the unit.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value
-
getSystem
-
setSystem
- Parameters:
value
- The identification of the system that provides the coded form of the unit.
-
getCodeElement
- Returns:
code
(A computer processable form of the unit in some unit representation system.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value
-
hasCodeElement
-
hasCode
-
setCodeElement
- Parameters:
value
-code
(A computer processable form of the unit in some unit representation system.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value
-
getCode
-
setCode
- Parameters:
value
- A computer processable form of the unit in some unit representation system.
-
listChildren
- Overrides:
listChildren
in classElement
-
getNamedProperty
public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getNamedProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getProperty
public Base[] getProperty(int hash, String name, boolean checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
public Base setProperty(int hash, String name, Base value) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
setProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
- Overrides:
setProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
removeChild
- Overrides:
removeChild
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
makeProperty
- Overrides:
makeProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getTypesForProperty
public String[] getTypesForProperty(int hash, String name) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getTypesForProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
addChild
-
fhirType
-
copy
-
copyValues
-
typedCopy
-
equalsDeep
- Overrides:
equalsDeep
in classElement
-
equalsShallow
- Overrides:
equalsShallow
in classElement
-
isEmpty
-
getVersion
- Specified by:
getVersion
in interfaceICoding
-
hasVersion
- Specified by:
hasVersion
in interfaceICoding
-
supportsVersion
- Specified by:
supportsVersion
in interfaceICoding
-
getDisplay
- Specified by:
getDisplay
in interfaceICoding
-
hasDisplay
- Specified by:
hasDisplay
in interfaceICoding
-
supportsDisplay
- Specified by:
supportsDisplay
in interfaceICoding
-
fromUcum
-