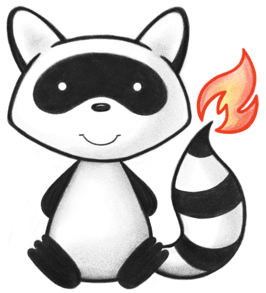
Package org.hl7.fhir.r5.model
Class Expression
java.lang.Object
org.hl7.fhir.r5.model.Base
org.hl7.fhir.r5.model.Element
org.hl7.fhir.r5.model.DataType
org.hl7.fhir.r5.model.Expression
- All Implemented Interfaces:
ca.uhn.fhir.model.api.IElement
,Serializable
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseDatatype
,org.hl7.fhir.instance.model.api.IBaseElement
,org.hl7.fhir.instance.model.api.IBaseHasExtensions
,org.hl7.fhir.instance.model.api.ICompositeType
Expression Type: A expression that is evaluated in a specified context and returns a value. The context of use of the expression must specify the context in which the expression is evaluated, and how the result of the expression is used.
- See Also:
-
Nested Class Summary
Nested classes/interfaces inherited from class org.hl7.fhir.r5.model.Base
Base.ProfileSource, Base.ValidationInfo, Base.ValidationMode, Base.ValidationReason
-
Field Summary
FieldsModifier and TypeFieldDescriptionprotected StringType
A brief, natural language description of the condition that effectively communicates the intended semantics.protected StringType
An expression in the specified language that returns a value.protected CodeType
The media type of the language for the expression.protected CodeType
A short name assigned to the expression to allow for multiple reuse of the expression in the context where it is defined.protected UriType
A URI that defines where the expression is found. -
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptioncopy()
void
copyValues
(Expression dst) boolean
equalsDeep
(Base other_) boolean
equalsShallow
(Base other_) fhirType()
getName()
getNamedProperty
(int _hash, String _name, boolean _checkValid) Base[]
getProperty
(int hash, String name, boolean checkValid) String[]
getTypesForProperty
(int hash, String name) boolean
boolean
boolean
boolean
boolean
boolean
boolean
hasName()
boolean
boolean
boolean
boolean
isEmpty()
protected void
listChildren
(List<Property> children) makeProperty
(int hash, String name) void
removeChild
(String name, Base value) setDescription
(String value) setDescriptionElement
(StringType value) setExpression
(String value) setExpressionElement
(StringType value) setLanguage
(String value) setLanguageElement
(CodeType value) setNameElement
(CodeType value) setProperty
(int hash, String name, Base value) setProperty
(String name, Base value) setReference
(String value) setReferenceElement
(UriType value) protected Expression
Methods inherited from class org.hl7.fhir.r5.model.DataType
copyValues, getTranslation, isTranslatable
Methods inherited from class org.hl7.fhir.r5.model.Element
addExtension, addExtension, addExtension, copyExtensions, copyNewExtensions, copyValues, getExtension, getExtensionByUrl, getExtensionFirstRep, getExtensionsByUrl, getExtensionsByUrl, getExtensionString, getExtensionString, getFHIRPublicationVersion, getId, getIdBase, getIdElement, getStandardsStatus, hasExtension, hasExtension, hasExtension, hasExtension, hasId, hasIdElement, isDisallowExtensions, noExtensions, removeExtension, setDisallowExtensions, setExtension, setId, setIdBase, setIdElement, setStandardsStatus
Methods inherited from class org.hl7.fhir.r5.model.Base
addDefinition, addFormatCommentsPost, addFormatCommentsPre, addValidationMessage, canHavePrimitiveValue, children, clearUserData, compareDeep, compareDeep, compareDeep, compareDeep, compareValues, compareValues, copyFormatComments, copyUserData, copyValues, dateTimeValue, equals, getChildByName, getChildValueByName, getFormatCommentsPost, getFormatCommentsPre, getNamedProperty, getUserData, getUserInt, getUserString, getValidationInfo, getValidationMessages, getXhtml, hasFormatComment, hasFormatCommentPost, hasFormatCommentPre, hasPrimitiveValue, hasType, hasUserData, hasValidated, hasValidationInfo, hasValidationMessages, isBooleanPrimitive, isCopyUserData, isDateTime, isMetadataBased, isPrimitive, isResource, listChildrenByName, listChildrenByName, primitiveValue, setCopyUserData, setUserData, setUserDataINN
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
getFormatCommentsPost, getFormatCommentsPre, getUserData, hasFormatComment, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseElement
getUserData, setUserData
-
Field Details
-
description
A brief, natural language description of the condition that effectively communicates the intended semantics. -
name
A short name assigned to the expression to allow for multiple reuse of the expression in the context where it is defined. -
language
The media type of the language for the expression. -
expression
An expression in the specified language that returns a value. -
reference
A URI that defines where the expression is found.
-
-
Constructor Details
-
Expression
public Expression()Constructor
-
-
Method Details
-
getDescriptionElement
- Returns:
description
(A brief, natural language description of the condition that effectively communicates the intended semantics.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value
-
hasDescriptionElement
-
hasDescription
-
setDescriptionElement
- Parameters:
value
-description
(A brief, natural language description of the condition that effectively communicates the intended semantics.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value
-
getDescription
- Returns:
- A brief, natural language description of the condition that effectively communicates the intended semantics.
-
setDescription
- Parameters:
value
- A brief, natural language description of the condition that effectively communicates the intended semantics.
-
getNameElement
- Returns:
name
(A short name assigned to the expression to allow for multiple reuse of the expression in the context where it is defined.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value
-
hasNameElement
-
hasName
-
setNameElement
- Parameters:
value
-name
(A short name assigned to the expression to allow for multiple reuse of the expression in the context where it is defined.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value
-
getName
- Returns:
- A short name assigned to the expression to allow for multiple reuse of the expression in the context where it is defined.
-
setName
- Parameters:
value
- A short name assigned to the expression to allow for multiple reuse of the expression in the context where it is defined.
-
getLanguageElement
- Returns:
language
(The media type of the language for the expression.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value
-
hasLanguageElement
-
hasLanguage
-
setLanguageElement
- Parameters:
value
-language
(The media type of the language for the expression.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value
-
getLanguage
- Returns:
- The media type of the language for the expression.
-
setLanguage
- Parameters:
value
- The media type of the language for the expression.
-
getExpressionElement
- Returns:
expression
(An expression in the specified language that returns a value.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value
-
hasExpressionElement
-
hasExpression
-
setExpressionElement
- Parameters:
value
-expression
(An expression in the specified language that returns a value.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value
-
getExpression
- Returns:
- An expression in the specified language that returns a value.
-
setExpression
- Parameters:
value
- An expression in the specified language that returns a value.
-
getReferenceElement
- Returns:
reference
(A URI that defines where the expression is found.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value
-
hasReferenceElement
-
hasReference
-
setReferenceElement
- Parameters:
value
-reference
(A URI that defines where the expression is found.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value
-
getReference
- Returns:
- A URI that defines where the expression is found.
-
setReference
- Parameters:
value
- A URI that defines where the expression is found.
-
listChildren
- Overrides:
listChildren
in classElement
-
getNamedProperty
public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getNamedProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getProperty
public Base[] getProperty(int hash, String name, boolean checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
public Base setProperty(int hash, String name, Base value) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
setProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
- Overrides:
setProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
removeChild
- Overrides:
removeChild
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
makeProperty
- Overrides:
makeProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getTypesForProperty
public String[] getTypesForProperty(int hash, String name) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getTypesForProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
addChild
-
fhirType
-
copy
-
copyValues
-
typedCopy
-
equalsDeep
- Overrides:
equalsDeep
in classElement
-
equalsShallow
- Overrides:
equalsShallow
in classElement
-
isEmpty
-