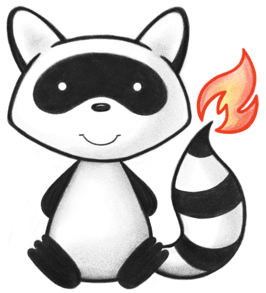
Package org.hl7.fhir.r5.model
Class Medication.MedicationIngredientComponent
java.lang.Object
org.hl7.fhir.r5.model.Base
org.hl7.fhir.r5.model.Element
org.hl7.fhir.r5.model.BackboneElement
org.hl7.fhir.r5.model.Medication.MedicationIngredientComponent
- All Implemented Interfaces:
ca.uhn.fhir.model.api.IElement
,Serializable
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseBackboneElement
,org.hl7.fhir.instance.model.api.IBaseElement
,org.hl7.fhir.instance.model.api.IBaseHasExtensions
,org.hl7.fhir.instance.model.api.IBaseHasModifierExtensions
- Enclosing class:
Medication
public static class Medication.MedicationIngredientComponent
extends BackboneElement
implements org.hl7.fhir.instance.model.api.IBaseBackboneElement
- See Also:
-
Nested Class Summary
Nested classes/interfaces inherited from class org.hl7.fhir.r5.model.Base
Base.ProfileSource, Base.ValidationInfo, Base.ValidationMode, Base.ValidationReason
-
Field Summary
FieldsModifier and TypeFieldDescriptionprotected BooleanType
Indication of whether this ingredient affects the therapeutic action of the drug.protected CodeableReference
The ingredient (substance or medication) that the ingredient.strength relates to.protected DataType
Specifies how many (or how much) of the items there are in this Medication.Fields inherited from class org.hl7.fhir.r5.model.BackboneElement
modifierExtension
-
Constructor Summary
ConstructorsConstructorDescriptionConstructorConstructor -
Method Summary
Modifier and TypeMethodDescriptioncopy()
void
boolean
equalsDeep
(Base other_) boolean
equalsShallow
(Base other_) fhirType()
boolean
getItem()
getNamedProperty
(int _hash, String _name, boolean _checkValid) Base[]
getProperty
(int hash, String name, boolean checkValid) String[]
getTypesForProperty
(int hash, String name) boolean
boolean
boolean
hasItem()
boolean
boolean
boolean
boolean
boolean
isEmpty()
protected void
listChildren
(List<Property> children) makeProperty
(int hash, String name) void
removeChild
(String name, Base value) setIsActive
(boolean value) setIsActiveElement
(BooleanType value) setItem
(CodeableReference value) setProperty
(int hash, String name, Base value) setProperty
(String name, Base value) setStrength
(DataType value) Methods inherited from class org.hl7.fhir.r5.model.BackboneElement
addModifierExtension, addModifierExtension, addModifierExtension, checkNoModifiers, copyExtensions, copyNewExtensions, copyValues, getExtensionByUrl, getExtensionsByUrl, getExtensionsByUrl, getExtensionValue, getModifierExtension, getModifierExtensionFirstRep, hasExtension, hasExtension, hasModifierExtension, removeExtension, setModifierExtension
Methods inherited from class org.hl7.fhir.r5.model.Element
addExtension, addExtension, addExtension, copyExtensions, copyNewExtensions, copyValues, getExtension, getExtensionByUrl, getExtensionFirstRep, getExtensionString, getExtensionString, getFHIRPublicationVersion, getId, getIdBase, getIdElement, getStandardsStatus, hasExtension, hasExtension, hasId, hasIdElement, isDisallowExtensions, noExtensions, setDisallowExtensions, setExtension, setId, setIdBase, setIdElement, setStandardsStatus
Methods inherited from class org.hl7.fhir.r5.model.Base
addDefinition, addFormatCommentsPost, addFormatCommentsPre, addValidationMessage, canHavePrimitiveValue, children, clearUserData, compareDeep, compareDeep, compareDeep, compareDeep, compareValues, compareValues, copyFormatComments, copyUserData, copyValues, dateTimeValue, equals, executeFunction, getChildByName, getChildValueByName, getFormatCommentsPost, getFormatCommentsPre, getNamedProperty, getUserData, getUserInt, getUserString, getValidationInfo, getValidationMessages, getXhtml, hasFormatComment, hasFormatCommentPost, hasFormatCommentPre, hasPrimitiveValue, hasType, hasUserData, hasValidated, hasValidationInfo, hasValidationMessages, isBooleanPrimitive, isCopyUserData, isDateTime, isMetadataBased, isPrimitive, isResource, listChildrenByName, listChildrenByName, primitiveValue, setCopyUserData, setUserData, setUserDataINN, setXhtml
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
getFormatCommentsPost, getFormatCommentsPre, getUserData, hasFormatComment, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseElement
getUserData, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseHasExtensions
addExtension, getExtension, hasExtension
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseHasModifierExtensions
addModifierExtension, getModifierExtension, hasModifierExtension
-
Field Details
-
item
The ingredient (substance or medication) that the ingredient.strength relates to. This is represented as a concept from a code system or described in another resource (Substance or Medication). -
isActive
Indication of whether this ingredient affects the therapeutic action of the drug. -
strength
Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.
-
-
Constructor Details
-
MedicationIngredientComponent
public MedicationIngredientComponent()Constructor -
MedicationIngredientComponent
Constructor
-
-
Method Details
-
getItem
- Returns:
item
(The ingredient (substance or medication) that the ingredient.strength relates to. This is represented as a concept from a code system or described in another resource (Substance or Medication).)
-
hasItem
-
setItem
- Parameters:
value
-item
(The ingredient (substance or medication) that the ingredient.strength relates to. This is represented as a concept from a code system or described in another resource (Substance or Medication).)
-
getIsActiveElement
- Returns:
isActive
(Indication of whether this ingredient affects the therapeutic action of the drug.). This is the underlying object with id, value and extensions. The accessor "getIsActive" gives direct access to the value
-
hasIsActiveElement
-
hasIsActive
-
setIsActiveElement
- Parameters:
value
-isActive
(Indication of whether this ingredient affects the therapeutic action of the drug.). This is the underlying object with id, value and extensions. The accessor "getIsActive" gives direct access to the value
-
getIsActive
- Returns:
- Indication of whether this ingredient affects the therapeutic action of the drug.
-
setIsActive
- Parameters:
value
- Indication of whether this ingredient affects the therapeutic action of the drug.
-
getStrength
- Returns:
strength
(Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.)
-
getStrengthRatio
- Returns:
strength
(Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.)- Throws:
org.hl7.fhir.exceptions.FHIRException
-
hasStrengthRatio
-
getStrengthCodeableConcept
- Returns:
strength
(Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.)- Throws:
org.hl7.fhir.exceptions.FHIRException
-
hasStrengthCodeableConcept
-
getStrengthQuantity
- Returns:
strength
(Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.)- Throws:
org.hl7.fhir.exceptions.FHIRException
-
hasStrengthQuantity
-
hasStrength
-
setStrength
- Parameters:
value
-strength
(Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.)
-
listChildren
- Overrides:
listChildren
in classBackboneElement
-
getNamedProperty
public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getNamedProperty
in classBackboneElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getProperty
public Base[] getProperty(int hash, String name, boolean checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getProperty
in classBackboneElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
public Base setProperty(int hash, String name, Base value) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
setProperty
in classBackboneElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
- Overrides:
setProperty
in classBackboneElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
removeChild
- Overrides:
removeChild
in classBackboneElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
makeProperty
- Overrides:
makeProperty
in classBackboneElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getTypesForProperty
public String[] getTypesForProperty(int hash, String name) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getTypesForProperty
in classBackboneElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
addChild
- Overrides:
addChild
in classBackboneElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
copy
- Specified by:
copy
in classBackboneElement
-
copyValues
-
equalsDeep
- Overrides:
equalsDeep
in classBackboneElement
-
equalsShallow
- Overrides:
equalsShallow
in classBackboneElement
-
isEmpty
- Specified by:
isEmpty
in interfaceorg.hl7.fhir.instance.model.api.IBase
- Overrides:
isEmpty
in classBackboneElement
-
fhirType
- Specified by:
fhirType
in interfaceorg.hl7.fhir.instance.model.api.IBase
- Overrides:
fhirType
in classBackboneElement
- Returns:
- the FHIR type name of the instance (not the java class name)
-