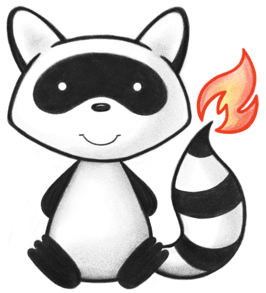
Enum Pointcut
- All Implemented Interfaces:
IPointcut
,Serializable
,Comparable<Pointcut>
Hook.value()
Hook pointcuts are divided into several broad categories:
- INTERCEPTOR_xxx: Hooks on the interceptor infrastructure itself
- CLIENT_xxx: Hooks on the HAPI FHIR Client framework
- SERVER_xxx: Hooks on the HAPI FHIR Server framework
- SUBSCRIPTION_xxx: Hooks on the HAPI FHIR Subscription framework
- STORAGE_xxx: Hooks on the storage engine
- VALIDATION_xxx: Hooks on the HAPI FHIR Validation framework
- JPA_PERFTRACE_xxx: Performance tracing hooks on the JPA server
-
Enum Constant Summary
Enum ConstantsEnum ConstantDescriptionCDS Hooks Prefetch Hook: Invoked after a failed CDS Hooks prefetch request.CDS Hooks Prefetch Hook: Invoked before a CDS Hooks prefetch request is made.CDS Hooks Prefetch Hook: Invoked after CDS Hooks prefetch request is completed successfully.Client Hook: This hook is called before an HTTP client request is sentClient Hook: This hook is called after an HTTP client request has completed, prior to returning the results to the calling code.Interceptor Framework Hook: This pointcut will be called once when a given interceptor is registeredPerformance Tracing Hook: This hook is invoked when a query involving an external index (e.g.Performance Tracing Hook: This hook is invoked when any informational messages generated by the SearchCoordinator are created.Performance Tracing Hook: This hook is invoked when a query has executed, and includes the raw SQL statements that were executed against the database.Performance Tracing Hook: This hook is invoked when a search has failed for any reason.Performance Tracing Hook: This hook is invoked when a search has failed for any reason.Performance Tracing Hook: This hook is invoked when a search has returned the very first result from the database.Performance Tracing Hook:Performance Tracing Hook: This hook is invoked when a search has completed.Performance Tracing Hook: Invoked when the storage engine is about to reuse the results of a previously cached search.Performance Tracing Hook: This hook is invoked when an individual search query SQL SELECT statement has completed and no more results are available from that query.Performance Tracing Hook: This hook is invoked when any warning messages generated by the SearchCoordinator are created.JPA Hook: This hook is invoked when a cross-partition reference is about to be stored in the database.MDM(EMPI) Hook: Invoked whenever a persisted resource (a resource that has just been stored in the database via a create/update/patch/etc.) has been matched against related resources and MDM links have been updated.MDM(EMPI) Hook: Invoked when a persisted resource (a resource that has just been stored in the database via a create/update/patch/etc.) enters the MDM module.MDM Clear Hook: This hook is invoked when an mdm clear operation is requested.MDM Create Link This hook is invoked after an MDM link is created, and changes have been persisted to the database.MDM Link History Hook: This hook is invoked after link histories are queried, but before the results are returned to the caller.MDM Merge Golden Resources This hook is invoked after 2 golden resources have been merged together and results persisted.MDM Not Duplicate/Unduplicate Hook: This hook is invoked after 2 golden resources with an existing link of "POSSIBLE_DUPLICATE" get unlinked/unduplicated.MDM Update Link This hook is invoked after an MDM link is updated, and changes have been persisted to the database.MDM Submit Hook: This hook is invoked whenever when mdm submit operation is requested.MDM_SUBMIT_PRE_MESSAGE_DELIVERY Hook: Invoked immediately before the delivery of a MESSAGE to the broker.Server Hook: This hook is called when a server CapabilityStatement is generated for returning to a client.Server Hook: This hook is invoked upon any exception being thrown within the server's request processing code.Server Hook: This method is called just before the actual implementing server method is invoked.Server Hook: This hook is invoked before an incoming request is processed.Server Hook: This method is immediately before the handling method is selected.Server Hook: This hook is called before any other processing takes place for each incoming request.Server Hook: This method is called when an OperationOutcome is being returned in response to a failure.Server Hook: This method is called after the server implementation method has been called, but before any attempt to stream the response back to the client, specifically for GraphQL requests (as these do not fit cleanly into the model provided bySERVER_OUTGOING_RESPONSE
).Server Hook: This method is called after the server implementation method has been called, but before any attempt to stream the response back to the client.Server Hook: This method is called when a stream writer is generated that will be used to stream a non-binary response to a client.Server Hook: This method is called upon any exception being thrown within the server's request processing code.Server Hook: This method is called after all processing is completed for a request, regardless of whether the request completed successfully or not.Server Hook: This method is called after all processing is completed for a request, but only if the request completes normally (i.e.Server Hook: This method is called when a resource provider method is registered and being bound by the HAPI FHIR Plain Server / RestfulServer.Binary Content Prefix Assigning Hook:Deprecated.Storage Hook: Invoked when a Bulk Export job is being processed.Storage Hook: Invoked when a resource is being deleted in a cascaded delete.Storage Hook: Invoked when a Bulk Export job is being kicked off.Storage Hook: Invoked when a partition has been created, typically meaning the$partition-management-create-partition
operation has been invoked.Storage Hook: Invoked when a partition has been deleted, typically meaning the$partition-management-delete-partition
operation has been invoked.Storage Hook: Invoked before FHIR operations to request the identification of the partition ID to be associated with the request being made.Storage Hook: Invoked before FHIR create operation to request the identification of the partition ID to be associated with the resource being created.Storage Hook: Invoked before any FHIR read/access/extended operation (e.g.Storage Hook: Invoked before any partition aware FHIR operation, when the selected partition has been identified (ie.Storage Hook: Invoked when a set of resources are about to be deleted and expunged via url likehttp://localhost/Patient?active=false&_expunge=true
.Storage Hook: Invoked when a batch of resource pids are about to be deleted and expunged via url likehttp://localhost/Patient?active=false&_expunge=true
.Storage Hook: Invoked when a Bulk Export job is being kicked off, but before any permission checks have been done.Storage Hook: Invoked when one or more resources may be returned to the user, whether as a part of a READ, a SEARCH, or even as the response to a CREATE/UPDATE, etc.Storage Hook: Invoked when the storage engine is about to check for the existence of a pre-cached search whose results match the given search parameters.Storage Hook: Invoked before a resource will be created, immediately before the transaction is committed (after all validation and other business rules have successfully completed, and any other database activity is complete.Storage Hook: Invoked before a resource will be deletedStorage Hook: Invoked before a resource will be updated, immediately before the transaction is committed (after all validation and other business rules have successfully completed, and any other database activity is complete.Storage Hook: Invoked when a search is starting, prior to creating a record for the search.Storage Hook: Invoked when one or more resources may be returned to the user, whether as a part of a READ, a SEARCH, or even as the response to a CREATE/UPDATE, etc.Storage Hook: Invoked before a batch job is persisted to the database.Storage Hook: Invoked before client-assigned id is created.Storage Hook: Invoked when a resource delete operation is about to fail due to referential integrity checks.Storage Hook: Invoked before an$expunge
operation on all data (expungeEverything) is called.Storage Hook: Invoked before a resource is about to be expunged via the$expunge
operation.Storage Hook: Invoked before a resource will be created, immediately before the resource is persisted to the database.Storage Hook: Invoked before a resource will be deleted, immediately before the resource is removed from the database.Storage Hook: Invoked before a resource will be updated, immediately before the resource is persisted to the database.Storage Hook: Invoked after all entries in a transaction bundle have been executedStorage Hook: Invoked when a FHIR transaction bundle is about to begin processing.Storage Hook: Invoked during a FHIR transaction, immediately after processing all write operations (i.e.Storage Hook: Invoked during a FHIR transaction, immediately before processing all write operations (i.e.Storage Hook: Invoked when a transaction has been rolled back as a result of aResourceVersionConflictException
, meaning that a database constraint has been violated.Subscription Hook: Invoked immediately after an active subscription is "registered".Subscription Hook: Invoked immediately after an active subscription is "registered".Subscription Hook: Invoked immediately after the delivery of a subscription, and right before any channel-specific hooks are invoked (e.g.Subscription Hook: Invoked immediately after the attempted delivery of a subscription, if the delivery failed.Subscription Hook: Invoked immediately after the delivery of MESSAGE subscription.Subscription Hook: Invoked whenever a persisted resource (a resource that has just been stored in the database via a create/update/patch/etc.) has been checked for whether any subscriptions were triggered as a result of the operation.Subscription Hook: Invoked immediately after the delivery of a REST HOOK subscription.Subscription Hook: Invoked immediately before the delivery of a subscription, and right before any channel-specific hooks are invoked (e.g.Subscription Hook: Invoked immediately before the delivery of a MESSAGE subscription.Subscription Hook: Invoked whenever a persisted resource (a resource that has just been stored in the database via a create/update/patch/etc.) is about to be checked for whether any subscriptions were triggered as a result of the operation.Subscription Hook: Invoked immediately before the delivery of a REST HOOK subscription.Subscription Hook: Invoked whenever a persisted resource was checked against all active subscriptions, and did not match any.Subscription Hook: Invoked any time that a resource is matched by an individual subscription, and is about to be queued for delivery.Subscription Hook: Invoked whenever a persisted resource has been modified and is being submitted to the subscription processing pipeline.Subscription Topic Hook: Invoked whenever a persisted resource (a resource that has just been stored in the database via a create/update/patch/etc.) has been checked for whether any subscription topics were triggered as a result of the operation.Subscription Topic Hook: Invoked whenever a persisted resource (a resource that has just been stored in the database via a create/update/patch/etc.) is about to be checked for whether any subscription topics were triggered as a result of the operation.This pointcut is used only for unit tests.This pointcut is used only for unit tests.Validation Hook: This hook is called after validation has completed, regardless of whether the validation was successful or failed. -
Method Summary
Modifier and TypeMethodDescriptionClass
<?> Class
<?> boolean
isShouldLogAndSwallowException
(Throwable theException) static Pointcut
Returns the enum constant of this type with the specified name.static Pointcut[]
values()
Returns an array containing the constants of this enum type, in the order they are declared.
-
Enum Constant Details
-
INTERCEPTOR_REGISTERED
Interceptor Framework Hook: This pointcut will be called once when a given interceptor is registered -
CLIENT_REQUEST
Client Hook: This hook is called before an HTTP client request is sentHooks may accept the following parameters:
- ca.uhn.fhir.rest.client.api.IHttpRequest - The details of the request
- ca.uhn.fhir.rest.client.api.IRestfulClient - The client object making the request
void
. -
CLIENT_RESPONSE
Client Hook: This hook is called after an HTTP client request has completed, prior to returning the results to the calling code. Hook methods may modify the response.Hooks may accept the following parameters:
- ca.uhn.fhir.rest.client.api.IHttpRequest - The details of the request
- ca.uhn.fhir.rest.client.api.IHttpResponse - The details of the response
- ca.uhn.fhir.rest.client.api.IRestfulClient - The client object making the request
- ca.uhn.fhir.rest.client.api.ClientResponseContext - Contains an IHttpRequest, an IHttpResponse, and an IRestfulClient and also allows the client to mutate the contained IHttpResponse
void
. -
SERVER_CAPABILITY_STATEMENT_GENERATED
Server Hook: This hook is called when a server CapabilityStatement is generated for returning to a client.This pointcut will not necessarily be invoked for every client request to the `/metadata` endpoint. If caching of the generated CapabilityStatement is enabled, a new CapabilityStatement will be generated periodically and this pointcut will be invoked at that time.
Hooks may accept the following parameters:
-
org.hl7.fhir.instance.model.api.IBaseConformance - The
CapabilityStatement
resource that will be returned to the client by the server. Interceptors may make changes to this resource. The parameter must be of typeIBaseConformance
, so it is the responsibility of the interceptor hook method code to cast to the appropriate version. - ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
CapabilityStatement
resource which will replace the one that was supplied to the interceptor, orvoid
to use the original one. If the interceptor chooses to modify theCapabilityStatement
that was supplied to the interceptor, it is fine for your hook method to returnvoid
ornull
. -
org.hl7.fhir.instance.model.api.IBaseConformance - The
-
SERVER_INCOMING_REQUEST_PRE_PROCESSED
Server Hook: This hook is called before any other processing takes place for each incoming request. It may be used to provide alternate handling for some requests, or to screen requests before they are handled, etc.Note that any exceptions thrown by this method will not be trapped by HAPI (they will be passed up to the server)
Hooks may accept the following parameters:
- jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment
- jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment
true
orvoid
if processing should continue normally. This is generally the right thing to do. If your interceptor is providing a response rather than letting HAPI handle the response normally, you must returnfalse
. In this case, no further processing will occur and no further interceptors will be called. -
SERVER_HANDLE_EXCEPTION
Server Hook: This hook is invoked upon any exception being thrown within the server's request processing code. This includes any exceptions thrown within resource provider methods (e.g.Search
andRead
methods) as well as any runtime exceptions thrown by the server itself. This also includes anyAuthenticationException
thrown.Hooks may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment
- jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment
- ca.uhn.fhir.rest.server.exceptions.BaseServerResponseException - The exception that was thrown
Implementations of this method may choose to ignore/log/count/etc exceptions, and return
true
orvoid
. In this case, processing will continue, and the server will automatically generate anOperationOutcome
. Implementations may also choose to provide their own response to the client. In this case, they should returnfalse
, to indicate that they have handled the request and processing should stop. -
SERVER_INCOMING_REQUEST_PRE_HANDLER_SELECTED
Server Hook: This method is immediately before the handling method is selected. Interceptors may make changes to the request that can influence which handler will ultimately be called.Hooks may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated at the time this hook is called.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment
- jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment
Hook methods may return
true
orvoid
if processing should continue normally. This is generally the right thing to do. If your interceptor is providing an HTTP response rather than letting HAPI handle the response normally, you must returnfalse
. In this case, no further processing will occur and no further interceptors will be called.Hook methods may also throw
AuthenticationException
if they would like. This exception may be thrown to indicate that the interceptor has detected an unauthorized access attempt. If thrown, processing will stop and an HTTP 401 will be returned to the client.- Since:
- 5.4.0
-
SERVER_INCOMING_REQUEST_POST_PROCESSED
Server Hook: This method is called just before the actual implementing server method is invoked.Hooks may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment
- jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment
Hook methods may return
true
orvoid
if processing should continue normally. This is generally the right thing to do. If your interceptor is providing an HTTP response rather than letting HAPI handle the response normally, you must returnfalse
. In this case, no further processing will occur and no further interceptors will be called.Hook methods may also throw
AuthenticationException
if they would like. This exception may be thrown to indicate that the interceptor has detected an unauthorized access attempt. If thrown, processing will stop and an HTTP 401 will be returned to the client. -
SERVER_INCOMING_REQUEST_PRE_HANDLED
Server Hook: This hook is invoked before an incoming request is processed. Note that this method is called after the server has begun preparing the response to the incoming client request. As such, it is not able to supply a response to the incoming request in the way that SERVER_INCOMING_REQUEST_PRE_PROCESSED andSERVER_INCOMING_REQUEST_POST_PROCESSED
are. At this point the request has already been passed to the handler so any changes (e.g. adding parameters) will not be considered. If you'd like to modify request parameters before they are passed to the handler, useSERVER_INCOMING_REQUEST_PRE_HANDLER_SELECTED
orSERVER_INCOMING_REQUEST_POST_PROCESSED
. If you are attempting to modify a search before it occurs, useSTORAGE_PRESEARCH_REGISTERED
.Hooks may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.rest.api.RestOperationTypeEnum - The type of operation that the FHIR server has determined that the client is trying to invoke
Hook methods must return
void
Hook methods method may throw a subclass of
BaseServerResponseException
, and processing will be aborted with an appropriate error returned to the client. -
SERVER_PROVIDER_METHOD_BOUND
Server Hook: This method is called when a resource provider method is registered and being bound by the HAPI FHIR Plain Server / RestfulServer.Hooks may accept the following parameters:
- ca.uhn.fhir.rest.server.method.BaseMethodBinding - The method binding.
Hook methods may modify the method binding, replace it, or return
null
to cancel the binding. -
SERVER_PRE_PROCESS_OUTGOING_EXCEPTION
Server Hook: This method is called upon any exception being thrown within the server's request processing code. This includes any exceptions thrown within resource provider methods (e.g.Search
andRead
methods) as well as any runtime exceptions thrown by the server itself. This hook method is invoked for each interceptor (until one of them returns a non-null
response or the end of the list is reached), after whichSERVER_HANDLE_EXCEPTION
is called for each interceptor.This may be used to add an OperationOutcome to a response, or to convert between exception types for any reason.
Implementations of this method may choose to ignore/log/count/etc exceptions, and return
null
. In this case, processing will continue, and the server will automatically generate anOperationOutcome
. Implementations may also choose to provide their own response to the client. In this case, they should return a non-null
, to indicate that they have handled the request and processing should stop.Hooks may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
-
java.lang.Throwable - The exception that was thrown. This will often be an instance of
BaseServerResponseException
but will not necessarily be one (e.g. it could be aNullPointerException
in the case of a bug being triggered. - jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment
- jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment
Hook methods may return a new exception to use for processing, or
null
if this interceptor is not trying to modify the exception. For example, if this interceptor has nothing to do with exception processing, it should always returnnull
. If this interceptor adds an OperationOutcome to the exception, it should return an exception. -
SERVER_OUTGOING_RESPONSE
Server Hook: This method is called after the server implementation method has been called, but before any attempt to stream the response back to the client. Interceptors may examine or modify the response before it is returned, or even prevent the response.Hooks may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
-
org.hl7.fhir.instance.model.api.IBaseResource - The resource that will be returned. This parameter may be
null
for some responses. - ca.uhn.fhir.rest.api.server.ResponseDetails - This object contains details about the response, including the contents. Hook methods may modify this object to change or replace the response.
- jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment
- jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment
Hook methods may return
true
orvoid
if processing should continue normally. This is generally the right thing to do. If your interceptor is providing a response rather than letting HAPI handle the response normally, you must returnfalse
. In this case, no further processing will occur and no further interceptors will be called.Hook methods may also throw
AuthenticationException
to indicate that the interceptor has detected an unauthorized access attempt. If thrown, processing will stop and an HTTP 401 will be returned to the client. -
SERVER_OUTGOING_WRITER_CREATED
Server Hook: This method is called when a stream writer is generated that will be used to stream a non-binary response to a client. Hooks may return a wrapped writer which adds additional functionality as needed.Hooks may accept the following parameters:
- java.io.Writer - The response writing Writer. Typically a hook will wrap this writer and layer additional functionality into the wrapping writer.
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
Hook methods should return a
Writer
instance that will be used to stream the response. Hook methods should not throw any exception.- Since:
- 5.0.0
-
SERVER_OUTGOING_GRAPHQL_RESPONSE
Server Hook: This method is called after the server implementation method has been called, but before any attempt to stream the response back to the client, specifically for GraphQL requests (as these do not fit cleanly into the model provided bySERVER_OUTGOING_RESPONSE
).Hooks may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- java.lang.String - The GraphQL query
- java.lang.String - The GraphQL response
- jakarta.servlet.http.HttpServletRequest - The servlet request, when running in a servlet environment
- jakarta.servlet.http.HttpServletResponse - The servlet response, when running in a servlet environment
Hook methods may return
true
orvoid
if processing should continue normally. This is generally the right thing to do. If your interceptor is providing a response rather than letting HAPI handle the response normally, you must returnfalse
. In this case, no further processing will occur and no further interceptors will be called.Hook methods may also throw
AuthenticationException
to indicate that the interceptor has detected an unauthorized access attempt. If thrown, processing will stop and an HTTP 401 will be returned to the client. -
SERVER_OUTGOING_FAILURE_OPERATIONOUTCOME
Server Hook: This method is called when an OperationOutcome is being returned in response to a failure. Hook methods may use this hook to modify the OperationOutcome being returned.Hooks may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- org.hl7.fhir.instance.model.api.IBaseOperationOutcome - The OperationOutcome resource that will be returned.
Hook methods must return
void
-
SERVER_PROCESSING_COMPLETED_NORMALLY
Server Hook: This method is called after all processing is completed for a request, but only if the request completes normally (i.e. no exception is thrown).This pointcut is called after the response has completely finished, meaning that the HTTP response to the client may or may not have already completely been returned to the client by the time this pointcut is invoked. Use caution if you have timing-dependent logic, since there is no guarantee about whether the client will have already moved on by the time your method is invoked. If you need a guarantee that your method is invoked before returning to the client, consider using
SERVER_OUTGOING_RESPONSE
instead.Hooks may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the request. This will be null if the server is not deployed to a RestfulServer environment.
This method must return
void
This method should not throw any exceptions. Any exception that is thrown by this method will be logged, but otherwise not acted upon (i.e. even if a hook method throws an exception, processing will continue and other interceptors will be called). Therefore it is considered a bug to throw an exception from hook methods using this pointcut.
-
SERVER_PROCESSING_COMPLETED
Server Hook: This method is called after all processing is completed for a request, regardless of whether the request completed successfully or not. It is called afterSERVER_PROCESSING_COMPLETED_NORMALLY
in the case of successful operations.Hooks may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the request. This will be null if the server is not deployed to a RestfulServer environment.
This method must return
void
This method should not throw any exceptions. Any exception that is thrown by this method will be logged, but otherwise not acted upon (i.e. even if a hook method throws an exception, processing will continue and other interceptors will be called). Therefore it is considered a bug to throw an exception from hook methods using this pointcut.
-
SUBSCRIPTION_RESOURCE_MODIFIED
Subscription Hook: Invoked whenever a persisted resource has been modified and is being submitted to the subscription processing pipeline. This method is called before the resource is placed on any queues for processing and executes synchronously during the resource modification operation itself, so it should return quickly.Hooks may accept the following parameters:
- ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - Hooks may modify this parameter. This will affect the checking process.
Hooks may return
void
or may return aboolean
. If the method returnsvoid
ortrue
, processing will continue normally. If the method returnsfalse
, subscription processing will not proceed for the given resource; -
SUBSCRIPTION_RESOURCE_MATCHED
Subscription Hook: Invoked any time that a resource is matched by an individual subscription, and is about to be queued for delivery.Hooks may make changes to the delivery payload, or make changes to the canonical subscription such as adding headers, modifying the channel endpoint, etc.
Hooks may accept the following parameters:- ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription
- ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage
- ca.uhn.fhir.jpa.searchparam.matcher.InMemoryMatchResult
Hooks may return
void
or may return aboolean
. If the method returnsvoid
ortrue
, processing will continue normally. If the method returnsfalse
, delivery will be aborted. -
SUBSCRIPTION_RESOURCE_DID_NOT_MATCH_ANY_SUBSCRIPTIONS
Subscription Hook: Invoked whenever a persisted resource was checked against all active subscriptions, and did not match any.Hooks may accept the following parameters:
- ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - Hooks should not modify this parameter as changes will not have any effect.
Hooks should return
void
. -
SUBSCRIPTION_BEFORE_DELIVERY
Subscription Hook: Invoked immediately before the delivery of a subscription, and right before any channel-specific hooks are invoked (e.g.SUBSCRIPTION_BEFORE_REST_HOOK_DELIVERY
.Hooks may make changes to the delivery payload, or make changes to the canonical subscription such as adding headers, modifying the channel endpoint, etc.
Hooks may accept the following parameters:- ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription
- ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage
Hooks may return
void
or may return aboolean
. If the method returnsvoid
ortrue
, processing will continue normally. If the method returnsfalse
, processing will be aborted. -
SUBSCRIPTION_AFTER_DELIVERY
Subscription Hook: Invoked immediately after the delivery of a subscription, and right before any channel-specific hooks are invoked (e.g.SUBSCRIPTION_AFTER_REST_HOOK_DELIVERY
.Hooks may accept the following parameters:
- ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription
- ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage
Hooks should return
void
. -
SUBSCRIPTION_AFTER_DELIVERY_FAILED
Subscription Hook: Invoked immediately after the attempted delivery of a subscription, if the delivery failed.Hooks may accept the following parameters:
- java.lang.Exception - The exception that caused the failure. Note this could be an exception thrown by a SUBSCRIPTION_BEFORE_DELIVERY or SUBSCRIPTION_AFTER_DELIVERY interceptor
- ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage - the message that triggered the exception
- java.lang.Exception
Hooks may return
void
or may return aboolean
. If the method returnsvoid
ortrue
, processing will continue normally, meaning that an exception will be thrown by the delivery mechanism. This typically means that the message will be returned to the processing queue. If the method returnsfalse
, processing will be aborted and no further action will be taken for the delivery. -
SUBSCRIPTION_AFTER_REST_HOOK_DELIVERY
Subscription Hook: Invoked immediately after the delivery of a REST HOOK subscription.When this hook is called, all processing is complete so this hook should not make any changes to the parameters.
Hooks may accept the following parameters:- ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription
- ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage
Hooks should return
void
. -
SUBSCRIPTION_BEFORE_REST_HOOK_DELIVERY
Subscription Hook: Invoked immediately before the delivery of a REST HOOK subscription.Hooks may make changes to the delivery payload, or make changes to the canonical subscription such as adding headers, modifying the channel endpoint, etc.
Hooks may accept the following parameters:- ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription
- ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage
Hooks may return
void
or may return aboolean
. If the method returnsvoid
ortrue
, processing will continue normally. If the method returnsfalse
, processing will be aborted. -
SUBSCRIPTION_AFTER_MESSAGE_DELIVERY
Subscription Hook: Invoked immediately after the delivery of MESSAGE subscription.When this hook is called, all processing is complete so this hook should not make any changes to the parameters.
Hooks may accept the following parameters:- ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription
- ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage
Hooks should return
void
. -
SUBSCRIPTION_BEFORE_MESSAGE_DELIVERY
Subscription Hook: Invoked immediately before the delivery of a MESSAGE subscription.Hooks may make changes to the delivery payload, or make changes to the canonical subscription such as adding headers, modifying the channel endpoint, etc. Furthermore, you may modify the outgoing message wrapper, for example adding headers via ResourceModifiedJsonMessage field.
Hooks may accept the following parameters:- ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription
- ca.uhn.fhir.jpa.subscription.model.ResourceDeliveryMessage
- ca.uhn.fhir.jpa.subscription.model.ResourceModifiedJsonMessage
Hooks may return
void
or may return aboolean
. If the method returnsvoid
ortrue
, processing will continue normally. If the method returnsfalse
, processing will be aborted. -
SUBSCRIPTION_BEFORE_PERSISTED_RESOURCE_CHECKED
Subscription Hook: Invoked whenever a persisted resource (a resource that has just been stored in the database via a create/update/patch/etc.) is about to be checked for whether any subscriptions were triggered as a result of the operation.Hooks may accept the following parameters:
- ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - Hooks may modify this parameter. This will affect the checking process.
Hooks may return
void
or may return aboolean
. If the method returnsvoid
ortrue
, processing will continue normally. If the method returnsfalse
, processing will be aborted. -
SUBSCRIPTION_AFTER_PERSISTED_RESOURCE_CHECKED
Subscription Hook: Invoked whenever a persisted resource (a resource that has just been stored in the database via a create/update/patch/etc.) has been checked for whether any subscriptions were triggered as a result of the operation.Hooks may accept the following parameters:
- ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - This parameter should not be modified as processing is complete when this hook is invoked.
Hooks should return
void
. -
SUBSCRIPTION_AFTER_ACTIVE_SUBSCRIPTION_REGISTERED
Subscription Hook: Invoked immediately after an active subscription is "registered". In HAPI FHIR, when a subscriptionHooks may make changes to the canonicalized subscription and this will have an effect on processing across this server. Note however that timing issues may occur, since the subscription is already technically live by the time this hook is called.
Hooks may accept the following parameters:- ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription
Hooks should return
void
. -
SUBSCRIPTION_AFTER_ACTIVE_SUBSCRIPTION_UNREGISTERED
Subscription Hook: Invoked immediately after an active subscription is "registered". In HAPI FHIR, when a subscriptionHooks may make changes to the canonicalized subscription and this will have an effect on processing across this server. Note however that timing issues may occur, since the subscription is already technically live by the time this hook is called.
No parameters are currently supported.Hooks should return
void
. -
STORAGE_CASCADE_DELETE
Storage Hook: Invoked when a resource is being deleted in a cascaded delete. This means that some other resource is being deleted, but per use request or other policy, the given resource (the one supplied as a parameter to this hook) is also being deleted.Hooks may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred. Note that this parameter may be null in contexts where the request is not known, such as while processing searches
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.jpa.util.DeleteConflictList - Contains the details about the delete conflicts that are being resolved via deletion. The source resource is the resource that will be deleted, and is a cascade because the target resource is already being deleted.
- org.hl7.fhir.instance.model.api.IBaseResource - The actual resource that is about to be deleted via a cascading delete
Hooks should return
void
. They may choose to throw an exception however, in which case the delete should be rolled back. -
SUBSCRIPTION_TOPIC_BEFORE_PERSISTED_RESOURCE_CHECKED
Subscription Topic Hook: Invoked whenever a persisted resource (a resource that has just been stored in the database via a create/update/patch/etc.) is about to be checked for whether any subscription topics were triggered as a result of the operation.Hooks may accept the following parameters:
- ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - Hooks may modify this parameter. This will affect the checking process.
Hooks may return
void
or may return aboolean
. If the method returnsvoid
ortrue
, processing will continue normally. If the method returnsfalse
, processing will be aborted. -
SUBSCRIPTION_TOPIC_AFTER_PERSISTED_RESOURCE_CHECKED
Subscription Topic Hook: Invoked whenever a persisted resource (a resource that has just been stored in the database via a create/update/patch/etc.) has been checked for whether any subscription topics were triggered as a result of the operation.Hooks may accept the following parameters:
- ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage - This parameter should not be modified as processing is complete when this hook is invoked.
Hooks should return
void
. -
STORAGE_PRE_INITIATE_BULK_EXPORT
Storage Hook: Invoked when a Bulk Export job is being kicked off, but before any permission checks have been done. This hook can be used to modify or update parameters as need be before authorization/permission checks are done.Hooks may accept the following parameters:
- ca.uhn.fhir.jpa.bulk.export.api.BulkDataExportOptions - The details of the job being kicked off
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred. Note that this parameter may be null in contexts where the request is not known, such as while processing searches
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
Hooks should return
void
, and can throw exceptions. -
STORAGE_INITIATE_BULK_EXPORT
Storage Hook: Invoked when a Bulk Export job is being kicked off. Hook methods may modify the request, or raise an exception to prevent it from being initiated. This hook is not guaranteed to be called before permission checks, and so anu implementers should be cautious of changing the options in ways that would affect permissions.Hooks may accept the following parameters:
- ca.uhn.fhir.jpa.bulk.export.api.BulkDataExportOptions - The details of the job being kicked off
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred. Note that this parameter may be null in contexts where the request is not known, such as while processing searches
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
Hooks should return
void
, and can throw exceptions. -
STORAGE_BULK_EXPORT_RESOURCE_INCLUSION
Storage Hook: Invoked when a Bulk Export job is being processed. If any hook method is registered for this pointcut, the hook method will be called once for each resource that is loaded for inclusion in a bulk export file. Hook methods may modify the resource object and this modification will affect the copy that is stored in the bulk export data file (but will not affect the original). Hook methods may also returnfalse
in order to request that the resource be filtered from the export.Hooks may accept the following parameters:
- ca.uhn.fhir.rest.api.server.bulk.BulkExportJobParameters - The details of the job being kicked off
- org.hl7.fhir.instance.model.api.IBaseResource - The resource that will be included in the file
Hooks methods may return
false
to indicate that the resource should be filtered out. Otherwise, hook methods should returntrue
.- Since:
- 6.8.0
-
STORAGE_PRE_DELETE_EXPUNGE
Storage Hook: Invoked when a set of resources are about to be deleted and expunged via url likehttp://localhost/Patient?active=false&_expunge=true
.Hooks may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred. Note that this parameter may be null in contexts where the request is not known, such as while processing searches
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- java.lang.String - Contains the url used to delete and expunge the resources
Hooks should return
void
. They may choose to throw an exception however, in which case the delete expunge will not occur. -
STORAGE_PRE_DELETE_EXPUNGE_PID_LIST
Storage Hook: Invoked when a batch of resource pids are about to be deleted and expunged via url likehttp://localhost/Patient?active=false&_expunge=true
.Hooks may accept the following parameters:
- java.lang.String - the name of the resource type being deleted
- java.util.List - the list of Long pids of the resources about to be deleted
- java.util.concurrent.atomic.AtomicLong - holds a running tally of all entities deleted so far. If the pointcut callback deletes any entities, then this parameter should be incremented by the total number of additional entities deleted.
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred. Note that this parameter may be null in contexts where the request is not known, such as while processing searches
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- java.lang.String - Contains the url used to delete and expunge the resources
Hooks should return
void
. They may choose to throw an exception however, in which case the delete expunge will not occur. -
STORAGE_PREACCESS_RESOURCES
Storage Hook: Invoked when one or more resources may be returned to the user, whether as a part of a READ, a SEARCH, or even as the response to a CREATE/UPDATE, etc.This hook is invoked when a resource has been loaded by the storage engine and is being returned to the HTTP stack for response. This is not a guarantee that the client will ultimately see it, since filters/headers/etc may affect what is returned but if a resource is loaded it is likely to be used. Note also that caching may affect whether this pointcut is invoked.
Hooks will have access to the contents of the resource being returned and may choose to make modifications. These changes will be reflected in returned resource but have no effect on storage.
Hooks may accept the following parameters:- ca.uhn.fhir.rest.api.server.IPreResourceAccessDetails - Contains details about the specific resources being returned.
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred. Note that this parameter may be null in contexts where the request is not known, such as while processing searches
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
Hooks should return
void
. -
STORAGE_PRECHECK_FOR_CACHED_SEARCH
Storage Hook: Invoked when the storage engine is about to check for the existence of a pre-cached search whose results match the given search parameters.Hooks may accept the following parameters:
- ca.uhn.fhir.jpa.searchparam.SearchParameterMap - Contains the details of the search being checked
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred. Note that this parameter may be null in contexts where the request is not known, such as while processing searches
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
Hooks may return
boolean
. If the hook method returnsfalse
, the server will not attempt to check for a cached search no matter what. -
STORAGE_PRESEARCH_REGISTERED
Storage Hook: Invoked when a search is starting, prior to creating a record for the search.Hooks may accept the following parameters:
- ca.uhn.fhir.rest.server.util.ICachedSearchDetails - Contains the details of the search that is being created and initialized. Interceptors may use this parameter to modify aspects of the search before it is stored and executed.
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred. Note that this parameter may be null in contexts where the request is not known, such as while processing searches
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.jpa.searchparam.SearchParameterMap - Contains the details of the search being checked. This can be modified.
- ca.uhn.fhir.interceptor.model.RequestPartitionId - The partition associated with the request (or null if the server is not partitioned)
Hooks should return
void
. -
STORAGE_PRESHOW_RESOURCES
Storage Hook: Invoked when one or more resources may be returned to the user, whether as a part of a READ, a SEARCH, or even as the response to a CREATE/UPDATE, etc.This hook is invoked when a resource has been loaded by the storage engine and is being returned to the HTTP stack for response. This is not a guarantee that the client will ultimately see it, since filters/headers/etc may affect what is returned but if a resource is loaded it is likely to be used. Note also that caching may affect whether this pointcut is invoked.
Hooks will have access to the contents of the resource being returned and may choose to make modifications. These changes will be reflected in returned resource but have no effect on storage.
Hooks may accept the following parameters:- ca.uhn.fhir.rest.api.server.IPreResourceShowDetails - Contains the resources that will be shown to the user. This object may be manipulated in order to modify the actual resources being shown to the user (e.g. for masking)
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred. Note that this parameter may be null in contexts where the request is not known, such as while processing searches
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
Hooks should return
void
. -
STORAGE_PRESTORAGE_RESOURCE_CREATED
Storage Hook: Invoked before a resource will be created, immediately before the resource is persisted to the database.Hooks will have access to the contents of the resource being created and may choose to make modifications to it. These changes will be reflected in permanent storage.
Hooks may accept the following parameters:- org.hl7.fhir.instance.model.api.IBaseResource
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0)
Hooks should return
void
. -
STORAGE_PRESTORAGE_CLIENT_ASSIGNED_ID
Storage Hook: Invoked before client-assigned id is created.Hooks will have access to the contents of the resource being created so that client-assigned ids can be allowed/denied. These changes will be reflected in permanent storage.
Hooks may accept the following parameters:- org.hl7.fhir.instance.model.api.IBaseResource
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
Hooks should return
void
. -
STORAGE_PRESTORAGE_RESOURCE_UPDATED
Storage Hook: Invoked before a resource will be updated, immediately before the resource is persisted to the database.Hooks will have access to the contents of the resource being updated (both the previous and new contents) and may choose to make modifications to the new contents of the resource. These changes will be reflected in permanent storage.
NO-OPS: If the client has submitted an update that does not actually make any changes (i.e. the resource they include in the PUT body is identical to the content that was already stored) the server may choose to ignore the update and perform a "NO-OP". In this case, this pointcut is still invoked, but
Hooks may accept the following parameters:STORAGE_PRECOMMIT_RESOURCE_UPDATED
will not be. Hook methods for this pointcut may make changes to the new contents of the resource being updated, and in this case the NO-OP will be cancelled andSTORAGE_PRECOMMIT_RESOURCE_UPDATED
will also be invoked.- org.hl7.fhir.instance.model.api.IBaseResource - The previous contents of the resource being updated
- org.hl7.fhir.instance.model.api.IBaseResource - The new contents of the resource being updated
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0)
Hooks should return
void
. -
STORAGE_PRESTORAGE_RESOURCE_DELETED
Storage Hook: Invoked before a resource will be deleted, immediately before the resource is removed from the database.Hooks will have access to the contents of the resource being deleted and may choose to make modifications related to it. These changes will be reflected in permanent storage.
Hooks may accept the following parameters:- org.hl7.fhir.instance.model.api.IBaseResource - The resource being deleted
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0)
Hooks should return
void
. -
STORAGE_PRECOMMIT_RESOURCE_CREATED
Storage Hook: Invoked before a resource will be created, immediately before the transaction is committed (after all validation and other business rules have successfully completed, and any other database activity is complete.Hooks will have access to the contents of the resource being created but should generally not make any changes as storage has already occurred. Changes will not be reflected in storage, but may be reflected in the HTTP response.
Hooks may accept the following parameters:- org.hl7.fhir.instance.model.api.IBaseResource
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0)
- Boolean - Whether this pointcut invocation was deferred or not(since 5.4.0)
- ca.uhn.fhir.rest.api.InterceptorInvocationTimingEnum - The timing at which the invocation of the interceptor took place. Options are ACTIVE and DEFERRED.
Hooks should return
void
. -
STORAGE_PRECOMMIT_RESOURCE_UPDATED
Storage Hook: Invoked before a resource will be updated, immediately before the transaction is committed (after all validation and other business rules have successfully completed, and any other database activity is complete.Hooks will have access to the contents of the resource being updated (both the previous and new contents) but should generally not make any changes as storage has already occurred. Changes will not be reflected in storage, but may be reflected in the HTTP response.
NO-OP note: See
Hooks may accept the following parameters:STORAGE_PRESTORAGE_RESOURCE_UPDATED
for a note on no-op updates when no changes are detected.- org.hl7.fhir.instance.model.api.IBaseResource - The previous contents of the resource
- org.hl7.fhir.instance.model.api.IBaseResource - The proposed new contents of the resource
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0)
- ca.uhn.fhir.rest.api.InterceptorInvocationTimingEnum - The timing at which the invocation of the interceptor took place. Options are ACTIVE and DEFERRED.
Hooks should return
void
. -
STORAGE_PRECOMMIT_RESOURCE_DELETED
Storage Hook: Invoked before a resource will be deletedHooks will have access to the contents of the resource being deleted but should not make any changes as storage has already occurred
Hooks may accept the following parameters:- org.hl7.fhir.instance.model.api.IBaseResource - The resource being deleted
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0)
- ca.uhn.fhir.rest.api.InterceptorInvocationTimingEnum - The timing at which the invocation of the interceptor took place. Options are ACTIVE and DEFERRED.
Hooks should return
void
. -
STORAGE_TRANSACTION_PROCESSING
Storage Hook: Invoked when a FHIR transaction bundle is about to begin processing. Hooks may choose to modify the bundle, and may affect processing by doing so.Hooks will have access to the original bundle, as well as all the deferred interceptor broadcasts related to the processing of the transaction bundle
Hooks may accept the following parameters:- org.hl7.fhir.instance.model.api.IBaseBundle - The Bundle being processed
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
Hooks should return
void
.- Since:
- 6.2.0
- See Also:
-
STORAGE_TRANSACTION_PROCESSED
Storage Hook: Invoked after all entries in a transaction bundle have been executedHooks will have access to the original bundle, as well as all the deferred interceptor broadcasts related to the processing of the transaction bundle
Hooks may accept the following parameters:- org.hl7.fhir.instance.model.api.IBaseBundle - The Bundle that wsa processed
- ca.uhn.fhir.rest.api.server.storage.DeferredInterceptorBroadcasts- A collection of pointcut invocations and their parameters which were deferred.
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0)
Hooks should return
void
.- See Also:
-
STORAGE_TRANSACTION_WRITE_OPERATIONS_PRE
Storage Hook: Invoked during a FHIR transaction, immediately before processing all write operations (i.e. immediately before a database transaction will be opened)Hooks may accept the following parameters:
- ca.uhn.fhir.interceptor.model.TransactionWriteOperationsDetails - Contains details about the transaction that is about to start
- ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0)
Hooks should return
void
. -
STORAGE_TRANSACTION_WRITE_OPERATIONS_POST
Storage Hook: Invoked during a FHIR transaction, immediately after processing all write operations (i.e. immediately after the transaction has been committed or rolled back). This hook will always be called ifSTORAGE_TRANSACTION_WRITE_OPERATIONS_PRE
has been called, regardless of whether the operation succeeded or failed.Hooks may accept the following parameters:
- ca.uhn.fhir.interceptor.model.TransactionWriteOperationsDetails - Contains details about the transaction that is about to start
- ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0)
Hooks should return
void
. -
STORAGE_PRESTORAGE_DELETE_CONFLICTS
Storage Hook: Invoked when a resource delete operation is about to fail due to referential integrity checks. Intended for use with ca.uhn.fhir.jpa.interceptor.CascadingDeleteInterceptor.Hooks will have access to the list of resources that have references to the resource being deleted.
Hooks may accept the following parameters:- ca.uhn.fhir.jpa.api.model.DeleteConflictList - The list of delete conflicts
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.rest.api.server.storage.TransactionDetails - The outer transaction details object (since 5.0.0)
Hooks should return
ca.uhn.fhir.jpa.delete.DeleteConflictOutcome
. If the interceptor returns a non-null result, the DeleteConflictOutcome can be used to indicate a number of times to retry. -
STORAGE_PRESTORAGE_EXPUNGE_RESOURCE
Storage Hook: Invoked before a resource is about to be expunged via the$expunge
operation.Hooks will be passed a reference to a counter containing the current number of records that have been deleted. If the hook deletes any records, the hook is expected to increment this counter by the number of records deleted.
Hooks may accept the following parameters:
- java.util.concurrent.atomic.AtomicInteger - The counter holding the number of records deleted.
- org.hl7.fhir.instance.model.api.IIdType - The ID of the resource that is about to be deleted
- org.hl7.fhir.instance.model.api.IBaseResource - The resource that is about to be deleted
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
Hooks should return void.
-
STORAGE_PRESTORAGE_EXPUNGE_EVERYTHING
Storage Hook: Invoked before an$expunge
operation on all data (expungeEverything) is called.Hooks will be passed a reference to a counter containing the current number of records that have been deleted. If the hook deletes any records, the hook is expected to increment this counter by the number of records deleted.
Hooks may accept the following parameters:- java.util.concurrent.atomic.AtomicInteger - The counter holding the number of records deleted.
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
Hooks should return void.
-
STORAGE_PARTITION_IDENTIFY_CREATE
Storage Hook: Invoked before FHIR create operation to request the identification of the partition ID to be associated with the resource being created. This hook will only be called if partitioning is enabled in the JPA server.Hooks may accept the following parameters:
- org.hl7.fhir.instance.model.api.IBaseResource - The resource that will be created and needs a tenant ID assigned.
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
Hooks must return an instance of
ca.uhn.fhir.interceptor.model.RequestPartitionId
.- See Also:
-
STORAGE_PARTITION_IDENTIFY_READ
Storage Hook: Invoked before any FHIR read/access/extended operation (e.g. read/vread, search, history, $reindex, etc.) operation to request the identification of the partition ID to be associated with the resource(s) being searched for, read, etc. Essentially any operations in the JPA server that are not creating a resource will use this pointcut. Creates will useSTORAGE_PARTITION_IDENTIFY_CREATE
.This hook will only be called if partitioning is enabled in the JPA server.
Hooks may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.interceptor.model.ReadPartitionIdRequestDetails - Contains details about what is being read
Hooks must return an instance of
ca.uhn.fhir.interceptor.model.RequestPartitionId
.- See Also:
-
STORAGE_PARTITION_IDENTIFY_ANY
Storage Hook: Invoked before FHIR operations to request the identification of the partition ID to be associated with the request being made.This hook is an alternative to
STORAGE_PARTITION_IDENTIFY_READ
andSTORAGE_PARTITION_IDENTIFY_CREATE
and can be used in cases where a partition interceptor does not need knowledge of the specific resources being accessed/read/written in order to determine the appropriate partition. If registered, then neither STORAGE_PARTITION_IDENTIFY_READ, nor STORAGE_PARTITION_IDENTIFY_CREATE will be called.This hook will only be called if partitioning is enabled in the JPA server.
Hooks may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
Hooks must return an instance of
ca.uhn.fhir.interceptor.model.RequestPartitionId
.- See Also:
-
STORAGE_PARTITION_CREATED
Storage Hook: Invoked when a partition has been created, typically meaning the$partition-management-create-partition
operation has been invoked.This hook will only be called if partitioning is enabled in the JPA server.
Hooks may accept the following parameters:
- ca.uhn.fhir.interceptor.model.RequestPartitionId - The partition ID that was selected
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
Hooks must return void.
-
STORAGE_PARTITION_DELETED
Storage Hook: Invoked when a partition has been deleted, typically meaning the$partition-management-delete-partition
operation has been invoked.This hook will only be called if partitioning is enabled in the JPA server.
Hooks may accept the following parameters:
- ca.uhn.fhir.interceptor.model.RequestPartitionId - The ID of the partition that was deleted.
Hooks must return void.
-
STORAGE_PARTITION_SELECTED
Storage Hook: Invoked before any partition aware FHIR operation, when the selected partition has been identified (ie. after theSTORAGE_PARTITION_IDENTIFY_CREATE
orSTORAGE_PARTITION_IDENTIFY_READ
hook was called. This allows a separate hook to register, and potentially make decisions about whether the request should be allowed to proceed.This hook will only be called if partitioning is enabled in the JPA server.
Hooks may accept the following parameters:
- ca.uhn.fhir.interceptor.model.RequestPartitionId - The partition ID that was selected
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.context.RuntimeResourceDefinition - The resource type being accessed, or null if no specific type is associated with the request.
Hooks must return void.
-
STORAGE_VERSION_CONFLICT
Storage Hook: Invoked when a transaction has been rolled back as a result of aResourceVersionConflictException
, meaning that a database constraint has been violated. This pointcut allows an interceptor to specify a resolution strategy other than simply returning the error to the client. This interceptor will be fired after the database transaction rollback has been completed.Hooks may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred. Note that this parameter may be null in contexts where the request is not known, such as while processing searches
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
Hooks should return
ca.uhn.fhir.jpa.api.model.ResourceVersionConflictResolutionStrategy
. Hooks should not throw any exception. -
VALIDATION_COMPLETED
Validation Hook: This hook is called after validation has completed, regardless of whether the validation was successful or failed. Typically this is used to modify validation results.Note on validation Pointcuts: The HAPI FHIR interceptor framework is a part of the client and server frameworks and not a part of the core FhirContext. Therefore this Pointcut is invoked by the
Hooks may accept the following parameters:
- org.hl7.fhir.instance.model.api.IBaseResource - The resource being validated, if a parsed version is available (null otherwise)
- java.lang.String - The resource being validated, if a raw version is available (null otherwise)
- ca.uhn.fhir.validation.ValidationResult - The outcome of the validation. Hooks methods should not modify this object, but they can return a new one.
ValidationResult
if they wish to override the validation results, or they may returnnull
orvoid
otherwise. -
MDM_BEFORE_PERSISTED_RESOURCE_CHECKED
MDM(EMPI) Hook: Invoked when a persisted resource (a resource that has just been stored in the database via a create/update/patch/etc.) enters the MDM module. The purpose of the pointcut is to permit a pseudo modification of the resource elements to influence the MDM linking process. Any modifications to the resource are not persisted.Hooks may accept the following parameters:
- org.hl7.fhir.instance.model.api.IBaseResource -
Hooks should return
void
. -
MDM_AFTER_PERSISTED_RESOURCE_CHECKED
MDM(EMPI) Hook: Invoked whenever a persisted resource (a resource that has just been stored in the database via a create/update/patch/etc.) has been matched against related resources and MDM links have been updated.Hooks may accept the following parameters:
- ca.uhn.fhir.rest.server.messaging.ResourceOperationMessage - This parameter should not be modified as processing is complete when this hook is invoked.
- ca.uhn.fhir.rest.server.TransactionLogMessages - This parameter is for informational messages provided by the MDM module during MDM processing.
- ca.uhn.fhir.mdm.model.mdmevents.MdmLinkEvent - Contains information about the change event, including target and golden resource IDs and the operation type.
Hooks should return
void
. -
MDM_POST_CREATE_LINK
MDM Create Link This hook is invoked after an MDM link is created, and changes have been persisted to the database.Hook may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request.
- ca.uhn.fhir.mdm.api.MdmLinkChangeEvent - Contains information about the link event, including target and golden resource IDs and the operation type.
Hooks should return
void
. -
MDM_POST_UPDATE_LINK
MDM Update Link This hook is invoked after an MDM link is updated, and changes have been persisted to the database.Hook may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request.
- ca.uhn.fhir.mdm.api.MdmLinkChangeEvent - Contains information about the link event, including target and golden resource IDs and the operation type.
Hooks should return
void
. -
MDM_POST_MERGE_GOLDEN_RESOURCES
MDM Merge Golden Resources This hook is invoked after 2 golden resources have been merged together and results persisted.Hook may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request.
- ca.uhn.fhir.mdm.model.mdmevents.MdmMergeEvent - Contains information about the from and to resources.
- ca.uhn.fhir.mdm.model.mdmevents.MdmTransactionContext - Contains information about the Transaction context, e.g. merge or link.
Hooks should return
void
. -
MDM_POST_LINK_HISTORY
MDM Link History Hook: This hook is invoked after link histories are queried, but before the results are returned to the caller.Hook may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed.
- ca.uhn.fhir.mdm.model.mdmevents.MdmHistoryEvent - An MDM History Event containing information about the requested golden resource ids and/or source ids input, and the returned link histories.
-
MDM_POST_NOT_DUPLICATE
MDM Not Duplicate/Unduplicate Hook: This hook is invoked after 2 golden resources with an existing link of "POSSIBLE_DUPLICATE" get unlinked/unduplicated.This hook accepts the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed.
- ca.uhn.fhir.mdm.model.mdmevents.MdmLinkEvent - the resulting final link between the 2 golden resources; now a NO_MATCH link.
-
MDM_CLEAR
MDM Clear Hook: This hook is invoked when an mdm clear operation is requested.This hook accepts the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed.
- ca.uhn.fhir.mdm.model.mdmevents.MdmClearEvent - the event containing information on the clear command, including the type filter (if any) and the batch size (if any).
-
MDM_SUBMIT
MDM Submit Hook: This hook is invoked whenever when mdm submit operation is requested. MDM submits can be invoked in multiple ways. Some of which accept asynchronous calling, and some of which do not.If the MDM Submit operation is asynchronous (typically because the Prefer: respond-async header has been provided) this hook will be invoked after the job is submitted, but before it has necessarily been executed.
If the MDM Submit operation is synchronous, this hook will be invoked immediately after the submit operation has been executed, but before the call is returned to the caller.
- On Patient Type. Can be synchronous or asynchronous.
- On Practitioner Type. Can be synchronous or asynchronous.
- On specific patient instances. Is always synchronous.
- On specific practitioner instances. Is always synchronous.
- On the server (ie, not on any resource) with or without a resource filter. Can be synchronous or asynchronous.
In all cases, this hook will take the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - An object containing details about the request that is about to be processed.
- ca.uhn.fhir.mdm.model.mdmevents.MdmSubmitEvent - An event with the Mdm Submit information (urls specifying paths that will be searched for MDM submit, as well as if this was an asynchronous request or not).
-
MDM_SUBMIT_PRE_MESSAGE_DELIVERY
MDM_SUBMIT_PRE_MESSAGE_DELIVERY Hook: Invoked immediately before the delivery of a MESSAGE to the broker.Hooks can make changes to the delivery payload. Furthermore, modification can be made to the outgoing message, for example adding headers or changing message key, which will be used for the subsequent processing.
Hooks should accept the following parameters:- ca.uhn.fhir.jpa.subscription.model.ResourceModifiedJsonMessage
-
JPA_RESOLVE_CROSS_PARTITION_REFERENCE
JPA Hook: This hook is invoked when a cross-partition reference is about to be stored in the database.This is an experimental API - It may change in the future, use with caution.
Hooks may accept the following parameters:
- ca.uhn.fhir.jpa.searchparam.extractor.CrossPartitionReferenceDetails - Contains details about the cross partition reference.
Hooks should return
void
. -
JPA_PERFTRACE_INFO
Performance Tracing Hook: This hook is invoked when any informational messages generated by the SearchCoordinator are created. It is typically used to provide logging or capture details related to a specific request.Note that this is a performance tracing hook. Use with caution in production systems, since calling it may (or may not) carry a cost.
Hooks may accept the following parameters:- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.jpa.model.search.StorageProcessingMessage - Contains the message
Hooks should return
void
. -
JPA_PERFTRACE_WARNING
Performance Tracing Hook: This hook is invoked when any warning messages generated by the SearchCoordinator are created. It is typically used to provide logging or capture details related to a specific request.Note that this is a performance tracing hook. Use with caution in production systems, since calling it may (or may not) carry a cost.
Hooks may accept the following parameters:- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.jpa.model.search.StorageProcessingMessage - Contains the message
Hooks should return
void
. -
JPA_PERFTRACE_SEARCH_FIRST_RESULT_LOADED
Performance Tracing Hook: This hook is invoked when a search has returned the very first result from the database. The timing on this call can be a good indicator of how performant a query is in general.Note that this is a performance tracing hook. Use with caution in production systems, since calling it may (or may not) carry a cost.
Hooks may accept the following parameters:- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being performed. Hooks should not modify this object.
Hooks should return
void
. -
JPA_PERFTRACE_SEARCH_SELECT_COMPLETE
Performance Tracing Hook: This hook is invoked when an individual search query SQL SELECT statement has completed and no more results are available from that query. Note that this doesn't necessarily mean that no more matching results exist in the database, since HAPI FHIR JPA batch loads results in to the query cache in chunks in order to provide predicable results without overloading memory or the database.Note that this is a performance tracing hook. Use with caution in production systems, since calling it may (or may not) carry a cost.
Hooks may accept the following parameters:- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being performed. Hooks should not modify this object.
Hooks should return
void
. -
JPA_PERFTRACE_SEARCH_FAILED
Performance Tracing Hook: This hook is invoked when a search has failed for any reason. When this pointcut is invoked, the search has completed unsuccessfully and will not be continued.Note that this is a performance tracing hook. Use with caution in production systems, since calling it may (or may not) carry a cost.
Hooks may accept the following parameters:- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being performed. Hooks should not modify this object.
Hooks should return
void
. -
JPA_PERFTRACE_SEARCH_PASS_COMPLETE
Performance Tracing Hook: This hook is invoked when a search has completed. When this pointcut is invoked, a pass in the Search Coordinator has completed successfully, but not all possible resources have been loaded yet so a future paging request may trigger a new task that will load further resources.Note that this is a performance tracing hook. Use with caution in production systems, since calling it may (or may not) carry a cost.
Hooks may accept the following parameters:- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being performed. Hooks should not modify this object.
Hooks should return
void
. -
JPA_PERFTRACE_INDEXSEARCH_QUERY_COMPLETE
Performance Tracing Hook: This hook is invoked when a query involving an external index (e.g. Elasticsearch) has completed. When this pointcut is invoked, an initial list of resource IDs has been generated which will be used as part of a subsequent database query.Note that this is a performance tracing hook. Use with caution in production systems, since calling it may (or may not) carry a cost.
Hooks may accept the following parameters:- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being performed. Hooks should not modify this object.
Hooks should return
void
. -
JPA_PERFTRACE_SEARCH_REUSING_CACHED
Performance Tracing Hook: Invoked when the storage engine is about to reuse the results of a previously cached search.Note that this is a performance tracing hook. Use with caution in production systems, since calling it may (or may not) carry a cost.
Hooks may accept the following parameters:
- ca.uhn.fhir.jpa.searchparam.SearchParameterMap - Contains the details of the search being checked
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred. Note that this parameter may be null in contexts where the request is not known, such as while processing searches
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
Hooks should return
void
. -
JPA_PERFTRACE_SEARCH_COMPLETE
Performance Tracing Hook: This hook is invoked when a search has failed for any reason. When this pointcut is invoked, a pass in the Search Coordinator has completed successfully, and all possible results have been fetched and loaded into the query cache.Note that this is a performance tracing hook. Use with caution in production systems, since calling it may (or may not) carry a cost.
Hooks may accept the following parameters:- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.jpa.model.search.SearchRuntimeDetails - Contains details about the search being performed. Hooks should not modify this object.
Hooks should return
void
. -
JPA_PERFTRACE_SEARCH_FOUND_ID
Performance Tracing Hook:This hook is invoked when a search has found an individual ID.
THIS IS AN EXPERIMENTAL HOOK AND MAY BE REMOVED OR CHANGED WITHOUT WARNING.
Note that this is a performance tracing hook. Use with caution in production systems, since calling it may (or may not) carry a cost.
Hooks may accept the following parameters:
- java.lang.Integer - The query ID
- java.lang.Object - The ID
Hooks should return
void
. -
JPA_PERFTRACE_RAW_SQL
Performance Tracing Hook: This hook is invoked when a query has executed, and includes the raw SQL statements that were executed against the database.Note that this is a performance tracing hook. Use with caution in production systems, since calling it may (or may not) carry a cost.
Hooks may accept the following parameters:
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.
- ca.uhn.fhir.rest.server.servlet.ServletRequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. This parameter is identical to the RequestDetails parameter above but will only be populated when operating in a RestfulServer implementation. It is provided as a convenience.
- ca.uhn.fhir.jpa.util.SqlQueryList - Contains details about the raw SQL queries.
Hooks should return
void
. -
STORAGE_BINARY_ASSIGN_BLOB_ID_PREFIX
@Deprecated(since="7.2.0 - Use STORAGE_BINARY_ASSIGN_BINARY_CONTENT_ID_PREFIX instead.") public static final Pointcut STORAGE_BINARY_ASSIGN_BLOB_ID_PREFIXDeprecated.Deprecated but still supported. Will eventually be removed.Please use Pointcut.STORAGE_BINARY_ASSIGN_BINARY_CONTENT_ID_PREFIX
Binary Blob Prefix Assigning Hook:Immediately before a binary blob is stored to its eventual data sink, this hook is called. This hook allows implementers to provide a prefix to the binary blob's ID. This is helpful in cases where you want to identify this blob for later retrieval outside of HAPI-FHIR. Note that allowable characters will depend on the specific storage sink being used.
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated.
- org.hl7.fhir.instance.model.api.IBaseBinary - The binary resource that is about to be stored.
Hooks should return
String
, which represents the full prefix to be applied to the blob. -
STORAGE_BINARY_ASSIGN_BINARY_CONTENT_ID_PREFIX
Binary Content Prefix Assigning Hook:Immediately before binary content is stored to its eventual data sink, this hook is called. This hook allows implementers to provide a prefix to the binary content's ID. This is helpful in cases where you want to identify this blob for later retrieval outside of HAPI-FHIR. Note that allowable characters will depend on the specific storage sink being used.
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of the servlet request. Note that the bean properties are not all guaranteed to be populated.
- org.hl7.fhir.instance.model.api.IBaseBinary - The binary resource that is about to be stored.
Hooks should return
String
, which represents the full prefix to be applied to the blob. -
STORAGE_PRESTORAGE_BATCH_JOB_CREATE
Storage Hook: Invoked before a batch job is persisted to the database.Hooks will have access to the content of the job being created and may choose to make modifications to it. These changes will be reflected in permanent storage.
Hooks may accept the following parameters:- ca.uhn.fhir.batch2.model.JobInstance
- ca.uhn.fhir.rest.api.server.RequestDetails - A bean containing details about the request that lead to the creation of the jobInstance.
Hooks should return
void
. -
CDS_HOOK_PREFETCH_REQUEST
CDS Hooks Prefetch Hook: Invoked before a CDS Hooks prefetch request is made. Hooks may accept the following parameters:- "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson" - The prefetch query, template and resolution strategy used for the request.
This parameter also contains a user data map
(String, Object)
, that allows data to be store between pointcut invocations of a prefetch request/response. - "ca.uhn.fhir.rest.api.server.cdshooks.CdsServiceRequestJson" - The CDS Hooks request that the prefetch is being made for
Hooks should return
void
. - "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson" - The prefetch query, template and resolution strategy used for the request.
This parameter also contains a user data map
-
CDS_HOOK_PREFETCH_RESPONSE
CDS Hooks Prefetch Hook: Invoked after CDS Hooks prefetch request is completed successfully. Hooks may accept the following parameters:- "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson" - The prefetch query and template and resolution strategy used for the request.
This parameter also contains a user data map
(String, Object)
, that allows data to be store between pointcut invocations of a prefetch request/response. - "ca.uhn.fhir.rest.api.server.cdshooks.CdsServiceRequestJson" - The CDS Hooks request that the prefetch is being made for
- "org.hl7.fhir.instance.model.api.IBaseResource" - The resource that is returned by the prefetch request
Hooks should return
void
. - "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson" - The prefetch query and template and resolution strategy used for the request.
This parameter also contains a user data map
-
CDS_HOOK_PREFETCH_FAILED
CDS Hooks Prefetch Hook: Invoked after a failed CDS Hooks prefetch request. Hooks may accept the following parameters:- "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson" - The prefetch query and template and resolution strategy used for the request.
This parameter also contains a user data map
(String, Object)
, that allows data to be store between pointcut invocations of a prefetch request/response. - "ca.uhn.fhir.rest.api.server.cdshooks.CdsServiceRequestJson" - The CDS Hooks request that the prefetch is being made for
- "java.lang.Exception" - The exception that caused the failure of the prefetch request
Hooks should return
void
. - "ca.uhn.hapi.fhir.cdshooks.api.json.prefetch.CdsHookPrefetchPointcutContextJson" - The prefetch query and template and resolution strategy used for the request.
This parameter also contains a user data map
-
TEST_RB
This pointcut is used only for unit tests. Do not use in production code as it may be changed or removed at any time. -
TEST_RO
This pointcut is used only for unit tests. Do not use in production code as it may be changed or removed at any time.
-
-
Method Details
-
values
Returns an array containing the constants of this enum type, in the order they are declared.- Returns:
- an array containing the constants of this enum type, in the order they are declared
-
valueOf
Returns the enum constant of this type with the specified name. The string must match exactly an identifier used to declare an enum constant in this type. (Extraneous whitespace characters are not permitted.)- Parameters:
name
- the name of the enum constant to be returned.- Returns:
- the enum constant with the specified name
- Throws:
IllegalArgumentException
- if this enum type has no constant with the specified nameNullPointerException
- if the argument is null
-
isShouldLogAndSwallowException
- Specified by:
isShouldLogAndSwallowException
in interfaceIPointcut
-
getReturnType
- Specified by:
getReturnType
in interfaceIPointcut
-
getBooleanReturnTypeForEnum
- Specified by:
getBooleanReturnTypeForEnum
in interfaceIPointcut
-
getParameterTypes
- Specified by:
getParameterTypes
in interfaceIPointcut
-