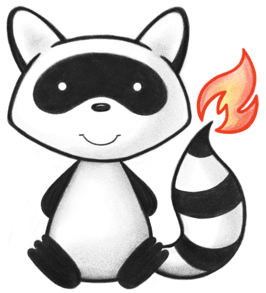
Class BaseServerResponseException
java.lang.Object
java.lang.Throwable
java.lang.Exception
java.lang.RuntimeException
ca.uhn.fhir.rest.server.exceptions.BaseServerResponseException
- All Implemented Interfaces:
Serializable
- Direct Known Subclasses:
AuthenticationException
,FhirClientConnectionException
,FhirClientInappropriateForServerException
,ForbiddenOperationException
,InternalErrorException
,InvalidRequestException
,InvalidResponseException
,MethodNotAllowedException
,NonFhirResponseException
,NotImplementedOperationException
,NotModifiedException
,PayloadTooLargeException
,ResourceGoneException
,ResourceNotFoundException
,ResourceVersionConflictException
,ResourceVersionNotSpecifiedException
,UnclassifiedServerFailureException
,UnprocessableEntityException
Base class for RESTful client and server exceptions. RESTful client methods will only throw exceptions which are subclasses of this exception type, and RESTful server methods should also only call
subclasses of this exception type.
HAPI provides a number of subclasses of BaseServerResponseException, and each one corresponds to a specific
HTTP status code. For example, if a IResourceProvider method throws
ResourceNotFoundException
, this is a signal to the server that an HTTP 404
should
be returned to the client.
See: A complete list of available exceptions is in the package summary.
If an exception doesn't exist for a condition you want to represent, let us know by filing an
issue in our tracker. You may also
use UnclassifiedServerFailureException
to represent any error code you want.
- See Also:
-
Constructor Summary
ConstructorsConstructorDescriptionBaseServerResponseException
(int theStatusCode, String theMessage) ConstructorBaseServerResponseException
(int theStatusCode, String... theMessages) ConstructorBaseServerResponseException
(int theStatusCode, String theMessage, Throwable theCause) ConstructorBaseServerResponseException
(int theStatusCode, String theMessage, Throwable theCause, IBaseOperationOutcome theBaseOperationOutcome) ConstructorBaseServerResponseException
(int theStatusCode, String theMessage, IBaseOperationOutcome theBaseOperationOutcome) ConstructorBaseServerResponseException
(int theStatusCode, Throwable theCause) ConstructorBaseServerResponseException
(int theStatusCode, Throwable theCause, IBaseOperationOutcome theBaseOperationOutcome) Constructor -
Method Summary
Modifier and TypeMethodDescriptionaddResponseHeader
(String theName, String theValue) Add a header which will be added to any responsesReturns theIBaseOperationOutcome
resource if any which was supplied in the response, ornull
In a RESTful client, this method will be populated with the body of the HTTP respone if one was provided by the server, ornull
otherwise.Returns a map containing any headers which should be added to the outgoing response.In a RESTful client, this method will be populated with the HTTP status code that was returned with the HTTP response.int
Returns the HTTP status code corresponding to this problemboolean
Does the exception have any headers which should be added to the outgoing response?boolean
This flag can be used to signal to server infrastructure that the message supplied to this exception (ie to the constructor) is considered trusted and is safe to return to the calling client.static BaseServerResponseException
newInstance
(int theStatusCode, String theMessage) setErrorMessageTrusted
(boolean theErrorMessageTrusted) This flag can be used to signal to server infrastructure that the message supplied to this exception (ie to the constructor) is considered trusted and is safe to return to the calling client.void
setOperationOutcome
(IBaseOperationOutcome theBaseOperationOutcome) Sets the BaseOperationOutcome resource associated with this exception.void
setResponseBody
(String theResponseBody) This method is currently only called internally by HAPI, it should not be called by user code.void
setResponseMimeType
(String theResponseMimeType) This method is currently only called internally by HAPI, it should not be called by user code.Methods inherited from class java.lang.Throwable
addSuppressed, fillInStackTrace, getCause, getLocalizedMessage, getMessage, getStackTrace, getSuppressed, initCause, printStackTrace, printStackTrace, printStackTrace, setStackTrace, toString
-
Constructor Details
-
BaseServerResponseException
Constructor- Parameters:
theStatusCode
- The HTTP status code corresponding to this problemtheMessage
- The message
-
BaseServerResponseException
Constructor- Parameters:
theStatusCode
- The HTTP status code corresponding to this problemtheMessages
- The messages
-
BaseServerResponseException
public BaseServerResponseException(int theStatusCode, String theMessage, IBaseOperationOutcome theBaseOperationOutcome) Constructor- Parameters:
theStatusCode
- The HTTP status code corresponding to this problemtheMessage
- The messagetheBaseOperationOutcome
- An BaseOperationOutcome resource to return to the calling client (in a server) or the BaseOperationOutcome that was returned from the server (in a client)
-
BaseServerResponseException
Constructor- Parameters:
theStatusCode
- The HTTP status code corresponding to this problemtheMessage
- The messagetheCause
- The cause
-
BaseServerResponseException
public BaseServerResponseException(int theStatusCode, String theMessage, Throwable theCause, IBaseOperationOutcome theBaseOperationOutcome) Constructor- Parameters:
theStatusCode
- The HTTP status code corresponding to this problemtheMessage
- The messagetheCause
- The underlying cause exceptiontheBaseOperationOutcome
- An BaseOperationOutcome resource to return to the calling client (in a server) or the BaseOperationOutcome that was returned from the server (in a client)
-
BaseServerResponseException
Constructor- Parameters:
theStatusCode
- The HTTP status code corresponding to this problemtheCause
- The underlying cause exception
-
BaseServerResponseException
public BaseServerResponseException(int theStatusCode, Throwable theCause, IBaseOperationOutcome theBaseOperationOutcome) Constructor- Parameters:
theStatusCode
- The HTTP status code corresponding to this problemtheCause
- The underlying cause exceptiontheBaseOperationOutcome
- An BaseOperationOutcome resource to return to the calling client (in a server) or the BaseOperationOutcome that was returned from the server (in a client)
-
-
Method Details
-
isErrorMessageTrusted
This flag can be used to signal to server infrastructure that the message supplied to this exception (ie to the constructor) is considered trusted and is safe to return to the calling client. -
setErrorMessageTrusted
This flag can be used to signal to server infrastructure that the message supplied to this exception (ie to the constructor) is considered trusted and is safe to return to the calling client. -
addResponseHeader
Add a header which will be added to any responses- Parameters:
theName
- The header nametheValue
- The header value- Returns:
- Returns a reference to
this
for easy method chaining - Since:
- 2.0
-
getAdditionalMessages
-
getOperationOutcome
Returns theIBaseOperationOutcome
resource if any which was supplied in the response, ornull
-
setOperationOutcome
Sets the BaseOperationOutcome resource associated with this exception. In server implementations, this is the OperartionOutcome resource to include with the HTTP response. In client implementations you should not call this method.- Parameters:
theBaseOperationOutcome
- The BaseOperationOutcome resource Sets the BaseOperationOutcome resource associated with this exception. In server implementations, this is the OperartionOutcome resource to include with the HTTP response. In client implementations you should not call this method.
-
getResponseBody
In a RESTful client, this method will be populated with the body of the HTTP respone if one was provided by the server, ornull
otherwise.In a restful server, this method is currently ignored.
-
setResponseBody
This method is currently only called internally by HAPI, it should not be called by user code. -
getResponseHeaders
Returns a map containing any headers which should be added to the outgoing response. This methos creates the map if none exists, so it will never returnnull
- Since:
- 2.0 (note that this method existed in previous versions of HAPI but the method
signature has been changed from
Map<String, String[]>
toMap<String, List<String>>
-
getResponseMimeType
In a RESTful client, this method will be populated with the HTTP status code that was returned with the HTTP response.In a restful server, this method is currently ignored.
-
setResponseMimeType
This method is currently only called internally by HAPI, it should not be called by user code. -
getStatusCode
Returns the HTTP status code corresponding to this problem -
hasResponseHeaders
Does the exception have any headers which should be added to the outgoing response?- Since:
- 2.0
- See Also:
-
newInstance
-